Angular 14+ standalone components

Greetings, everyone, and welcome to this article about Angular 14+ standalone components. Standalone components represent a novel addition to Angular, enabling the creation of reusable components that don't require an NgModule declaration. This approach enhances code modularity, efficiency, and facilitates sharing across projects.
What exactly are standalone components?
Standalone components are components flagged as "standalone." This specific marker informs the Angular compiler that these components don't need to be declared within an NgModule. While standalone components can import other standalone components and NgModules, they cannot export any dependencies.
Advantages of leveraging standalone components:
Modularity: Standalone components can be employed in isolation, eliminating the necessity for an NgModule. This streamlines your codebase, making it more modular and reusable.
Efficiency: Standalone components support tree-shaking, which means Angular only loads the components used in your application, enhancing performance.
Sharing: Standalone components can be shared across different Angular applications, simplifying the creation of reusable components for multiple projects.
Creating a standalone component is straightforward:
To create a standalone component, simply mark it with the standalone flag, as shown below:
@Component({
selector: 'my-standalone-component',
standalone: true,
})
export class MyStandaloneComponent {
// ...
}
Once created, you can import and utilize your standalone component in your application like any other component.
Utilizing standalone components within NgModule-based applications:
Standalone components can also be incorporated into NgModule-based applications. To do this, simply import the standalone component into your NgModule, like so:
@NgModule({
declarations: [
AppComponent,
MyStandaloneComponent,
],
imports: [
BrowserModule,
],
bootstrap: [AppComponent],
})
export class AppModule {}
Once imported into your NgModule, you can use your standalone component just like any other component in your application.
In conclusion:
Standalone components are a potent addition to Angular 14+, promoting code modularity, efficiency, and sharing. If you're developing new Angular components, it's recommended to use the standalone flag whenever applicable.
Additional tips for working with standalone components:
Use standalone components for small, reusable elements. For larger components, consider using an NgModule.
Combine standalone components with existing NgModule-based libraries or dependencies to leverage the Angular ecosystem.
Think about using Angular Elements to export your standalone components as custom elements. This simplifies their usage in other web applications.
I trust this article has been informative. Should you have any questions, please don't hesitate to leave a comment below.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
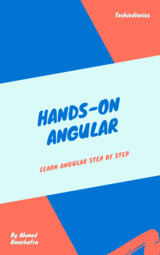