Angular 15 ReplaySubject

ReplaySubject is a type of subject, a special type of observable in RxJS, that stores multiple values from the observable sequence and replays them to new subscribers. It is similar to a behavior subject, but it can store more than one value and replay them to new subscribers.
Here is an example of how to use a ReplaySubject in an Angular 15 service:
import { Injectable } from '@angular/core';
import { ReplaySubject } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class MyService {
private myReplaySubject = new ReplaySubject<string>(2);
constructor() { }
addValue(value: string) {
this.myReplaySubject.next(value);
}
getValues() {
return this.myReplaySubject.asObservable();
}
}
In this example, we have a service with a ReplaySubject called myReplaySubject. The ReplaySubject is initialized with a buffer size of 2, which means it will store the last 2 values that have been passed to it. The service has two methods: addValue, which allows you to add a value to the ReplaySubject, and getValues, which returns the observable of the ReplaySubject.
Here is an example of how you can use this service in a component:
import { Component } from '@angular/core';
import { MyService } from './my.service';
@Component({
selector: 'app-my-component',
template: `
<p *ngFor="let value of values"></p>
`
})
export class MyComponent {
values: any;
constructor(private myService: MyService) {
this.values = this.myService.getValues();
}
addValue() {
this.myService.addValue('new value');
}
}
In this component, we inject the MyService and use the getValues method to get the observable of the ReplaySubject. We then bind the observable to the template using the ngFor directive. The component also has a method called addValue that adds a new value to the ReplaySubject by calling the addValue method of the service.
When the component is initialized, the ReplaySubject will be empty, so no values will be displayed in the template. When the addValue method is called, the value 'new value' will be added to the ReplaySubject and displayed in the template. If the addValue method is called again, the ReplaySubject will have a buffer size of 2, so it will store the last 2 values and replay them to new subscribers.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Hands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
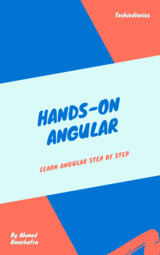