Asynchronous pipes in Angular: A Deeper dive

Angular 17 is a powerful front-end framework that provides developers with a myriad of tools for building dynamic and responsive web applications. One of its key features is the use of pipes, which allow you to transform and format data in your templates. Among these pipes, asynchronous pipes are particularly crucial when dealing with asynchronous operations and observables.
In this article, we'll take a deeper dive into asynchronous pipes in Angular 17. We'll explore what they are, why they're essential, and how to effectively use them in your Angular 17 applications.
Understanding Asynchronous Operations
Before we delve into asynchronous pipes, let's briefly recap what asynchronous operations are. In web development, asynchronous operations refer to tasks that can occur independently of the main program flow. Common examples include making HTTP requests, handling user input, and dealing with timers. These operations may take an unknown amount of time to complete, and you don't want them to block the user interface while waiting for their results.
Angular applications often rely on asynchronous operations, such as fetching data from a server, to provide real-time and interactive experiences. Asynchronous pipes are the perfect tool for handling data emitted from these asynchronous operations seamlessly.
What Are Asynchronous Pipes?
An asynchronous pipe is a special type of pipe in Angular that is designed to work with observables and promises. Observables are a core concept in Angular, representing streams of data over time. Asynchronous pipes subscribe to these observables and automatically update the view when new data is emitted.
Here's a breakdown of how asynchronous pipes work:
Subscription: When you use an asynchronous pipe in your template, it subscribes to an observable or promise.
Data Transformation: As new data is emitted over time, the asynchronous pipe automatically transforms and formats the data according to your template's requirements.
View Updates: The transformed data is then seamlessly displayed in the view, ensuring that your UI is always up-to-date with the latest values emitted by the asynchronous operation.
Why Asynchronous Pipes Are Essential
Asynchronous pipes offer several key advantages in Angular development:
Simplified Code: They eliminate the need for manual subscription and unsubscription, reducing the amount of boilerplate code and potential memory leaks.
Automatic Updates: Asynchronous pipes ensure that your view always reflects the most recent data emitted by an observable or promise, providing a real-time user experience.
Improved Readability: They make your templates cleaner and more readable by moving the logic for handling asynchronous data to the template itself.
Error Handling: Asynchronous pipes handle errors gracefully and allow you to provide fallback values or error messages in your templates.
How to Use Asynchronous Pipes
Using asynchronous pipes in Angular is straightforward. Here's a basic example:
<div *ngIf="userData$ | async as user">
<h1>Welcome, !</h1>
</div>
In this example:
- userData$ is an observable that emits user data.
- The *ngIf directive ensures that the content is displayed only when the observable emits data.
- The async pipe subscribes to userData$, automatically handling updates.
- The as user part assigns the emitted data to the variable user, which can then be used within the template.
Advanced Usage
Asynchronous pipes can be used in more complex scenarios, such as combining multiple observables, handling errors, or using the async pipe within structural directives like *ngFor or *ngSwitch.
Conclusion
Asynchronous pipes in Angular 17 are a fundamental tool for handling asynchronous data streams elegantly and efficiently. By simplifying code, automating updates, and improving readability, they contribute to creating responsive and user-friendly Angular applications. Whether you're working with HTTP requests, user input, or any other asynchronous operation, mastering asynchronous pipes is essential for building robust Angular applications.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
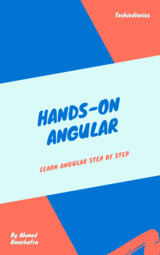