Angular 17 Material Autocomplete Multiselect Step-by-Step

Angular Material is a UI component library that provides a variety of reusable components for building Angular applications. One of these components is the autocomplete, which allows users to select from a list of options as they type. The autocomplete can also be used to create a multiselect, which allows users to select multiple options.
In this blog post, we will walk through the steps on how to implement an Angular 17 Material autocomplete multiselect.
Step 1: Install the Angular Material package:
You need to install and configure Angular Material in your Angular 17 project. The process is easy and straightforward.
Step2: Import the necessary modules into your Angular app:
import { MatAutocompleteModule } from '@angular/material/autocomplete';
import { MatChipListModule } from '@angular/material/chips';
import { MatFormFieldModule } from '@angular/material/form-field';
Create a component for your autocomplete multiselect:
import { Component, OnInit } from '@angular/core';
import { FormControl } from '@angular/forms';
import { Observable } from 'rxjs';
import { map, startWith } from 'rxjs/operators';
@Component({
selector: 'my-autocomplete-multiselect',
templateUrl: './autocomplete-multiselect.component.html',
styleUrls: ['./autocomplete-multiselect.component.css']
})
export class AutocompleteMultiselectComponent implements OnInit {
myControl = new FormControl();
options: Observable<string[]>;
constructor() { }
ngOnInit() {
this.options = this.myControl.valueChanges.pipe(
startWith(''),
map(value => {
const filterValue = value.toLowerCase();
return this.allOptions.filter(option => option.toLowerCase().includes(filterValue));
})
);
}
allOptions = ['Apple', 'Banana', 'Orange', 'Pear', 'Grape'];
selectedOptions: string[] = [];
addOption(option: string) {
this.selectedOptions.push(option);
this.myControl.setValue(this.selectedOptions);
}
removeOption(option: string) {
this.selectedOptions = this.selectedOptions.filter(item => item !== option);
this.myControl.setValue(this.selectedOptions);
}
}
Create a template for your autocomplete multiselect:
<mat-form-field appearance="outline">
<mat-label>Autocomplete multiselect</mat-label>
<input matInput type="text" [formControl]="myControl" [matAutocomplete]="auto">
<mat-autocomplete #auto="matAutocomplete">
<mat-option *ngFor="let option of options | async" (click)="addOption(option)">
</mat-option>
</mat-autocomplete>
</mat-form-field>
<mat-chip-list>
<mat-chip *ngFor="let option of selectedOptions" (remove)="removeOption(option)">
<mat-icon matChipRemove>cancel</mat-icon>
</mat-chip>
</mat-chip-list>
Add your component to your Angular app's template and start it up:
<my-autocomplete-multiselect></my-autocomplete-multiselect>
Step-by-step explanation:
Step 1: Install Angular Material Package
In the initial step, we begin by installing the Angular Material package. This package serves as a comprehensive resource containing all the essential components and styles needed for constructing Angular Material-based applications.
Step 2: Import Necessary Modules
For the second step, we proceed to import crucial modules into our Angular application. These modules are indispensable for harnessing the functionalities of the autocomplete and chip list components, which are pivotal to our multiselect implementation.
Step 3: Create Autocomplete Multiselect Component
In the third step, we embark on crafting a dedicated component tailored for our autocomplete multiselect feature. Within this component, we house the intricate logic responsible for dynamically filtering available options and managing the addition and removal of selected items.
Step 4: Design Autocomplete Multiselect Template
The subsequent step involves the creation of a template specifically designed for our autocomplete multiselect component. This template will elegantly present the autocomplete input element alongside a visually appealing chip list that showcases the currently selected options.
Step 5: Integrate Component into App Template
Lastly, we seamlessly integrate our custom component into our Angular application's overall template structure. This integration ensures that our autocomplete multiselect component harmoniously blends with the rest of the user interface. Subsequently, we initiate the application to set everything into motion.
Following these meticulous steps, we successfully bring to life an Angular Material autocomplete multiselect feature. This versatile component can be further tailored to meet our precise needs, whether it involves adding supplementary functionalities or applying unique styling to match the application's aesthetics.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
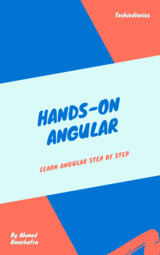