Building a Password Strength Meter in Angular 17

Implementing a password strength meter in Angular 17 is a valuable addition to any web application that requires user authentication. This tutorial will guide you through a detailed step-by-step process to create a password strength meter using Angular 17 and the zxcvbn
library.
Step 1: Set Up Your Angular 17 Environment
First, ensure you have Node.js and npm installed on your system. Then, install the Angular 17 CLI globally by running the following command in your terminal:
npm install -g @angular/cli
Step 2: Create a New Angular 17 Project
Open your terminal and create a new Angular 17 project by running the following command:
ng new password-strength-meter
cd password-strength-meter
Step 3: Install zxcvbn
Next, install the zxcvbn
library, which we'll use to calculate password strength. Run the following command in your project directory:
npm install zxcvbn
Step 4: Generate a Password Strength Component
Use the Angular 17 CLI to generate a new component for the password strength meter:
ng generate component password-strength
Step 5: Implement Password Strength Logic
Navigate to the newly created password-strength.component.ts
file in your password-strength
component directory. Add the following code to calculate the password strength:
import { Component, Input } from '@angular/core';
import * as zxcvbn from 'zxcvbn';
@Component({
selector: 'app-password-strength',
templateUrl: './password-strength.component.html',
styleUrls: ['./password-strength.component.css']
})
export class PasswordStrengthComponent {
@Input() password: string = '';
getPasswordStrength(): number {
const result = zxcvbn(this.password);
return result.score;
}
}
Step 6: Create the Password Strength Template
Open the password-strength.component.html
file and add the following code:
<div [ngClass]="{ 'weak': getPasswordStrength() < 3, 'strong': getPasswordStrength() >= 3 }">
<div class="strength-meter" [style.width]="getPasswordStrength() * 25 + '%'"></div>
</div>
Step 7: Style the Password Strength Meter
Open the password-strength.component.css
file and add the following styles:
.strength-meter {
height: 10px;
background-color: #ddd;
}
.weak .strength-meter {
background-color: #ff6961; /* Red for weak passwords */
}
.strong .strength-meter {
background-color: #77dd77; /* Green for strong passwords */
}
Step 8: Use the Password Strength Component
Now, you can use the app-password-strength
component in any other component by passing the password as an input.
Example Usage:
<app-password-strength [password]="userPassword"></app-password-strength>
Conclusion
Congratulations! You've successfully implemented a password strength meter in your Angular 17 application using the zxcvbn
library. This feature enhances the security of your application by providing users with feedback on the strength of their passwords. Feel free to customize the styling and further enhance the functionality based on your application's requirements.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Create Angular 17 Project Building a Password Strength Meter in Angular 17 Angular 17 Password Show/Hide with Eye Icon Angular 17 tutoriel Angular Select Change Event Angular iframe Angular FormArray setValue() and patchValue() Angular Find Substring in String Send File to API in Angular 17 EventEmitter Parent to Child Communication Create an Angular Material button with an icon and text Input change event in Angular 17 Find an element by ID in Angular 17 Find an element by ID from another component in Angular 17 Find duplicate objects in an array in JavaScript and Angular What is new with Angular 17 Style binding to text-decoration in Angular Remove an item from an array in Angular Remove a component in Angular Delete a component in Angular Use TypeScript enums in Angular templates Set the value of an individual reactive form fields Signal-based components in Angular 17 Angular libraries for Markdown: A comprehensive guide Angular libraries for cookies: A comprehensive guide Build an Angular 14 CRUD Example & Tutorial Angular 9 Components: Input and Output Angular 13 selectors Picture-in-Picture with JavaScript and Angular 10 Jasmine Unit Testing for Angular 12 Angular 9 Tutorial By Example: REST CRUD APIs & HTTP GET Requests with HttpClient Angular 10/9 Elements Tutorial by Example: Building Web Components Angular 10/9 Router Tutorial: Learn Routing & Navigation by Example Angular 10/9 Router CanActivate Guards and UrlTree Parsed Routes Angular 10/9 JWT Authentication Tutorial with Example Style Angular 10/9 Components with CSS and ngStyle/ngClass Directives Upload Images In TypeScript/Node & Angular 9/Ionic 5: Working with Imports, Decorators, Async/Await and FormData Angular 9/Ionic 5 Chat App: Unsubscribe from RxJS Subjects, OnDestroy/OnInit and ChangeDetectorRef Adding UI Guards, Auto-Scrolling, Auth State, Typing Indicators and File Attachments with FileReader to your Angular 9/Ionic 5 Chat App Private Chat Rooms in Angular 9/Ionic 5: Working with TypeScript Strings, Arrays, Promises, and RxJS Behavior/Replay Subjects Building a Chat App with TypeScript/Node.js, Ionic 5/Angular 9 & PubNub/Chatkit Chat Read Cursors with Angular 9/Ionic 5 Chat App: Working with TypeScript Variables/Methods & Textarea Keydown/Focusin Events Add JWT REST API Authentication to Your Node.js/TypeScript Backend with TypeORM and SQLite3 Database Building Chat App Frontend UI with JWT Auth Using Ionic 5/Angular 9 Install Angular 10 CLI with NPM and Create a New Example App with Routing Styling An Angular 10 Example App with Bootstrap 4 Navbar, Jumbotron, Tables, Forms and Cards Integrate Bootstrap 4/jQuery with Angular 10 and Styling the UI With Navbar and Table CSS Classes Angular 10/9 Tutorial and Example: Build your First Angular App Angular 9/8 ngIf Tutorial & Example Angular 10 New Features Create New Angular 9 Workspace and Application: Using Build and Serve Angular 10 Release Date: Angular 10 Will Focus on Ivy Artifacts and Libraries Support HTML5 Download Attribute with TypeScript and Angular 9 Angular 9.1+ Local Direction Query API: getLocaleDirection Example Angular 9.1 displayBlock CLI Component Generator Option by Example Angular 15 Basics Tutorial by Example Angular 9/8 ngFor Directive: Render Arrays with ngFor by Example Responsive Image Breakpoints Example with CDK's BreakpointObserver in Angular 9/8 Angular 9/8 DOM Queries: ViewChild and ViewChildren Example The Angular 9/8 Router: Route Parameters with Snapshot and ParamMap by Example Angular 9/8 Nested Routing and Child Routes by Example Angular 9 Examples: 2 Ways To Display A Component (Selector & Router) Angular 9/8 Tutorial: Http POST to Node/Express.js Example Angular 9/8 Feature and Root Modules by Example Angular 9/8 with PHP: Consuming a RESTful CRUD API with HttpClient and Forms Angular 9/8 with PHP and MySQL Database: REST CRUD Example & Tutorial Unit Testing Angular 9/8 Apps Tutorial with Jasmine & Karma by Example Angular 9 Web Components: Custom Elements & Shadow DOM Angular 9 Renderer2 with Directives Tutorial by Example Build Progressive Web Apps (PWA) with Angular 9/8 Tutorial and Example Angular 9 Internationalization/Localization with ngx-translate Tutorial and Example Create Angular 9 Calendar with ngx-bootstrap datepicker Example and Tutorial Multiple File Upload with Angular 9 FormData and PHP by Example Angular 9/8 Reactive Forms with Validation Tutorial by Example Angular 9/8 Template Forms Tutorial: Example Authentication Form (ngModel/ngForm/ngSubmit) Angular 9/8 JAMStack By Example Angular HttpClient v9/8 — Building a Service for Sending API Calls and Fetching Data Styling An Angular 9/8/7 Example App with Bootstrap 4 Navbar, Jumbotron, Tables, Forms and CardsHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
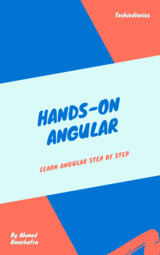