Angular Injection Context: What is it and how to use it

Dependency Injection (DI) is a pivotal design pattern that empowers developers to decouple their code, facilitating easier reuse of classes and services. In the context of Angular, DI plays a crucial role in supplying dependencies to components, directives, pipes, and other injectable classes, promoting a more modular and maintainable codebase.
Let's delve deeper into the Angular DI system and explore the various facets of the injection context, the places where DI can be employed, and best practices for harnessing its power effectively.
Understanding the Angular Injection Context
The Angular injection context is the runtime environment where the DI system operates, allowing the injection of dependencies into your classes and functions. It is accessible in several key places, which include:
Constructor of an Injectable Class: Dependencies can be injected directly into the constructor of an injectable class.
Factory Function for an InjectionToken: When using an InjectionToken, you can specify a factory function that provides the necessary dependency.
Factory Function for the useFactory Property of a Provider: Providers in Angular can utilize the useFactory property, which employs a factory function to supply dependencies.
Initializer for a Field in an Injectable Class: You can initialize a field within an injectable class, ensuring that it receives the required dependency.
Function Passed to runInInjectionContext: The runInInjectionContext function enables the execution of code within an injection context, ensuring that dependencies are available for injection.
To inject dependencies into your code within these contexts, the inject() function comes into play. The inject()
function accepts the token of the dependency you intend to inject as its sole argument, ensuring that Angular's DI system can seamlessly provide the required dependencies.
Practical Application of Dependency Injection
Let's explore how you can practically utilize the power of Angular's DI system:
Injecting Dependencies into Constructor
In the following example, we demonstrate how to inject the HttpClient service into a component's constructor:
import { Component, HttpClient } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private http: HttpClient) {}
ngOnInit() {
// Utilize the HttpClient service to make HTTP requests.
}
}
The Angular DI system automatically injects the HttpClient service into the AppComponent component during instantiation.
Injecting Dependencies into Functions
You can also employ the inject()
function to inject dependencies into functions that you define. In the following example, we create a function, makeHttpRequest()
, which injects the HttpClient service and uses it to make an HTTP request:
import { HttpClient } from '@angular/core';
function makeHttpRequest(http: HttpClient): Observable<any> {
return http.get('https://example.com/api/users');
}
This makeHttpRequest()
function can be used wherever you need to make an HTTP request within your Angular application.
Injecting Dependencies within Components
Here's an example of how to use the makeHttpRequest()
function in a component's ngOnInit()
method:
import { Component, HttpClient } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
users: Observable<any>;
constructor(private http: HttpClient) {}
ngOnInit() {
this.users = makeHttpRequest(this.http);
}
}
By integrating the makeHttpRequest()
function, you can dynamically fetch data and assign it to the users property.
Best Practices for Utilizing the Angular Injection Context
To make the most of the Angular injection context, consider the following best practices:
Inject Dependencies Only within an Injection Context: The
inject()
function should only be employed within an injection context. Attempting to use it outside of such a context will result in an error.Avoid Injecting Dependencies into Asynchronous Callbacks: Be cautious when injecting dependencies into functions that are passed to asynchronous callbacks. The injection context is not accessible in asynchronous callbacks, which may lead to code-breaking errors.
Use runInInjectionContext for Special Cases: In scenarios where you need to inject dependencies into code that typically does not run within an injection context, the runInInjectionContext function comes in handy. It enables you to execute code within an injection context, ensuring that dependencies are available for injection.
Embrace the Power of Angular's Dependency Injection
The Angular injection context is a formidable tool that facilitates the creation of clean, reusable, and maintainable code. By mastering the use of the injection context, you can harness the full potential of Angular's Dependency Injection system. Whether you are working on small-scale projects or complex applications, understanding how to leverage the injection context empowers you to build more modular and efficient Angular applications.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
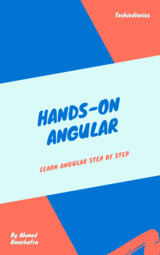