Import standalone components in Angular 14
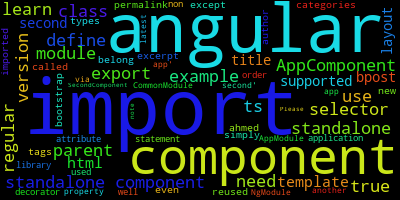
In this example, we'll learn about how to define and import angular 14 standalone components.
With Angular 14 version, we have new types of components called standalone components--they are simply regular components except that they don't belong to any parent module. They can be imported and reused and you can even use them to bootstrap the angular application.
How can standalone components be used from a library?
In regular, non-standalone components, we need to import the parent module.
How to import standalone components in Angular 14
In order to define a standalone component, you need to use the standalone
attribute in the decorator of the component. After that, you can use the imports
statement in the component as well. For example:
@Component({
standalone: true,
imports: [CommonModule],
selector: 'app',
template: `./app.component.html`,
})
export class AppComponent {}
With Angular 14, you can import the component in your module via the imports
property which wasn't supported before.
You can also import it into another component which also wasn't supported before the latest version. For example:
@NgModule({
imports: [AppComponent]
})
export class AppModule {}
@Component({
standalone: true,
imports: [AppComponent],
selector: 'second',
template: `./second.component.html`,
})
export class SecondComponent {}
Please note that the second component should also be a standalone component.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
How to send authorization header in Angular 14 Angular 14 Tutorial By Example: REST API & HttpClient GET Angular 14 route title and custom strategy Angular 14 inject example: reactive decorator Angular 14 inject How to enable CORS in Angular 14 Add Tailwind CSS to Angular 14 apps Import standalone components in Angular 14 Generate standalone components in Angular 14 Add Bootstrap to Angular 14 example Angular CLI 14 Render HTML with Angular and innerHtmlHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
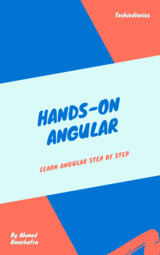