Routing and Navigation in Blazor WebAssembly
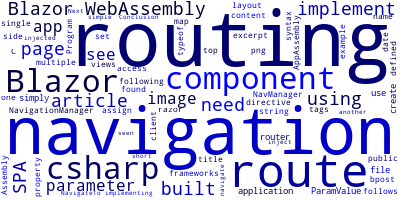
In this article, we'll see how to implement routing and navigation in your SPA application built with Blazor WebAssembly.
In SPA frameworks, you need to use client-side routing and navigation to create apps with multiple views.
Routing In Blazor WebAssembly
You can map a route to a Blazor component by using the @page directive at the top of your file. For example:
@page "/a-route"
You can assign more than one route to a single component:
@page "/route"
@page "/route/{ParamValue}"
To access the parameter, you can simply create a C# property with the name of the parameter as follows:
[Parameter]
public string? ParamValue { get; set; }
The routes are defined in Blazor components but the router component can be found in the App.razor
file:
<Router AppAssembly="@typeof(Program).Assembly">
Blazor Navigation
After implementing routing in your app, you'll also need navigation.
Just like routing, navigation is also simple. You simply need to use NavigationManager
which needs to be injected in your component using the following syntax:
@inject NavigationManager NavManager
Next, you can navigate to another component using the following syntax:
NavManager.NavigateTo("route/1");
Conclusion
In this short article, we've seen how you can implement routing and navigation in your SPA built using Blazor WebAssembly.
-
Date: