Angular 15 BehaviorSubject

BehaviorSubject is a type of subject, a special type of observable in RxJS, that keeps track of the current value and emits it to new subscribers. It is similar to a regular subject, but it also stores the latest value that has been passed to it. This is useful when you want to provide a default value for a subject or when you need to access the latest value of the subject.
Here is an example of how to use a BehaviorSubject in an Angular 15 service:
import { Injectable } from '@angular/core';
import { BehaviorSubject } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class MyService {
private myBehaviorSubject = new BehaviorSubject<string>('default value');
constructor() { }
setValue(value: string) {
this.myBehaviorSubject.next(value);
}
getValue() {
return this.myBehaviorSubject.asObservable();
}
}
In this example, we have a service with a BehaviorSubject called myBehaviorSubject. The BehaviorSubject is initialized with a default value of 'default value'. The service has two methods: setValue, which allows you to update the value of the BehaviorSubject, and getValue, which returns the observable of the BehaviorSubject.
Here is an example of how you can use this service in an Angular 15 component:
import { Component } from '@angular/core';
import { MyService } from './my.service';
@Component({
selector: 'app-my-component',
template: `
<p></p>
`
})
export class MyComponent {
myValue: any;
constructor(private myService: MyService) {
this.myValue = this.myService.getValue();
}
updateValue() {
this.myService.setValue('new value');
}
}
In this component, we inject the MyService and use the getValue method to get the observable of the BehaviorSubject. We then bind the observable to the template using the async pipe. The component also has a method called updateValue that updates the value of the BehaviorSubject by calling the setValue method of the service.
When the component is initialized, the value of the BehaviorSubject will be 'default value', which will be displayed in the template. When the updateValue method is called, the value of the BehaviorSubject will be updated to 'new value', and the template will update to display the new value.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Hands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
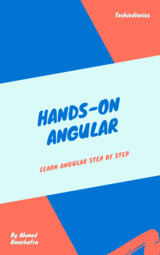