Angular 16 provideRouter Example: Use Standalone Components with Angular 16 Router

In this example we will learn about Angular 16 routing using provideRouter and standalone components.
We finally witnessed the arrival of standalone components in Angular v15.
The addition of @NgModule as optional transforms how we design routes and routing definitions.
So how can we establish routing in Angular 16 with standalone components?
We must first bootstrap the Angular application. Let's begin with a simple App
component that is bootstrapped using bootstrapApplication
:
import { Component } from '@angular/core';
import { bootstrapApplication } from '@angular/platform-browser';
@Component({
selector: 'my-app',
standalone: true,
template: `
Angular 16 provideRouter example
`,
})
export class App {}
bootstrapApplication(App);
Previously, we had to call the RouterModule.forRoot()
function and pass in our routing configurations.
So, how can we transition to the new standalone design while keeping our routing?
Suppose we have the following routes:
import { Route } from '@angular/router';
import { ProductsComponent } from '../products.component';
import { ProductComponent } from './product.component';
export const routes: Route[] = [
{
path: '',
component: CustomersComponent,
},
{
path: ':id',
component: CustomerComponent,
},
{
path: '**',
redirectTo: '',
},
];
These need to be added to a routes.ts
file.
Using provideRouter
to import routes
We must now import a new function provideRouter
and feed it to the bootstrapApplication
providers as follows:
import { Component } from '@angular/core';
import { provideRouter } from '@angular/router';
import { bootstrapApplication } from '@angular/platform-browser';
import { routes } from './routes';
@Component({
selector: 'my-app',
standalone: true,
template: `
Angular 16 provideRouter example
`,
})
export class App {}
bootstrapApplication(App, {
providers: [provideRouter(routes)],
});
That's OK for the time being, but we need a <router-outlet>
to render our components!
Import RouterOutlet
We'll need to import the RouterOutlet
directive and add it to the component imports metadata because we're utilizing standalone components:
import { Component } from '@angular/core';
import { provideRouter, RouterOutlet } from '@angular/router';
import { bootstrapApplication } from '@angular/platform-browser';
import { routes } from './routes';
@Component({
selector: 'my-app',
standalone: true,
imports: [RouterOutlet],
template: `
<router-outlet></router-outlet>
`,
})
export class App {}
bootstrapApplication(App, {
providers: [provideRouter(routes)],
});
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular 16 Signals - mutate & update examples Node.js v20.6.0: Introducing Built-in .env File Support Angular 16 Get Routing Parameters with @Input Angular 16 Required Input with Example Angular 16 provideRouter Example: Use Standalone Components with Angular 16 Router Add Jest Support to Angular 16 Angular 16 Inject HttpClient Angular 16 Get the Current Route Example Converting Signals to Observables in Angular 16Hands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
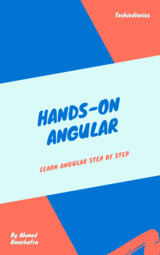