Angular 17 Material Autocomplete Get Selected Value Example

Angular Material's autocomplete component empowers users to make selections from a dynamic list of options while typing. Its versatility extends to accommodating various scenarios, such as picking a country from an extensive list or querying products within a catalog.
Within this blog post, our focus will be on elucidating the process of extracting the chosen value from an Angular Material autocomplete component. This guidance serves as a foundation that can be further expanded and tailored to meet your specific application needs.
Step 1: Install the Angular Material package:
You need to install and configure Angular Material in your Angular 17 project. The process is easy and straightforward.
Step 2: Import the necessary modules into your Angular app:
import { MatAutocompleteModule } from '@angular/material/autocomplete';
import { MatFormFieldModule } from '@angular/material/form-field';
Step 3: Create a component for your autocomplete:
import { Component, OnInit } from '@angular/core';
import { FormControl } from '@angular/forms';
@Component({
selector: 'my-autocomplete',
templateUrl: './autocomplete.component.html',
styleUrls: ['./autocomplete.component.css']
})
export class AutocompleteComponent implements OnInit {
myControl = new FormControl();
options = ['Apple', 'Banana', 'Orange', 'Pear', 'Grape'];
selectedOption: string;
ngOnInit() {
this.myControl.valueChanges.subscribe((value) => {
this.selectedOption = value;
});
}
}
Step 4: Create a template for your autocomplete:
<mat-form-field appearance="outline">
<mat-label>Autocomplete</mat-label>
<input matInput type="text" [formControl]="myControl" [matAutocomplete]="auto">
<mat-autocomplete #auto="matAutocomplete">
<mat-option *ngFor="let option of options" [value]="option">
</mat-option>
</mat-autocomplete>
</mat-form-field>
Step 5: Add your component to your Angular app's template and start it up:
<my-autocomplete></my-autocomplete>
Getting the selected value:
To get the selected value of the autocomplete, you can simply access the selectedOption variable. For example, you could use the following code to display the selected value in a mat-label:
<mat-label>Selected option: </mat-label>
You can also use the selected value to populate another form control or to perform other actions in your Angular app.
Conclusion
In this article, we've guided you through the process of retrieving the selected value from an Angular Material autocomplete component. While the example presented here is foundational, it serves as a starting point that you can adapt and extend to align with your particular requirements and customization preferences.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
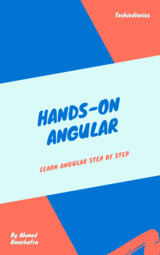