Send File to API in Angular 17

Sending files to an API using Angular involves creating an HTML form to select the file, handling the file selection event, constructing a multipart form data object, and making an HTTP POST request to the API. Here's a step-by-step guide:
- Create an HTML form: In your Angular component's HTML template, create an
<input type="file">
element to allow users to select a file. Use the(change)
event binding to handle the file selection.
<input type="file" (change)="onFileSelected($event)">
- Handle the file selection event: In your component's TypeScript code, define a method
onFileSelected
that receives the file selection event. This method will extract the selected file information and prepare it for upload.
onFileSelected(event: Event) {
const selectedFile = event.target['files'][0];
// Process the selected file
}
- Construct multipart form data: Create a new FormData object to represent the multipart form data. This object will hold the file data along with any other form data you might need to send.
const formData = new FormData();
formData.append('file', selectedFile, selectedFile.name);
// Add other form data if needed
- Make an HTTP POST request: Use Angular's HttpClient service to make an HTTP POST request to the API endpoint. Include the FormData object as the request body.
this.http.post<any>(apiUrl, formData, { headers: { 'Content-Type': 'multipart/form-data' } })
.subscribe(response => {
// Handle successful response
}, error => {
// Handle error response
});
- Handle the response: In the
subscribe
callback, handle the response from the API. If the response is successful, you can proceed with the uploaded file or perform any necessary actions. If there's an error, handle it appropriately.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Create Angular 17 Project Building a Password Strength Meter in Angular 17 Angular 17 Password Show/Hide with Eye Icon Angular 17 tutoriel Angular Select Change Event Angular iframe Angular FormArray setValue() and patchValue() Angular Find Substring in String Send File to API in Angular 17 EventEmitter Parent to Child Communication Create an Angular Material button with an icon and text Input change event in Angular 17 Find an element by ID in Angular 17 Find an element by ID from another component in Angular 17 Find duplicate objects in an array in JavaScript and Angular What is new with Angular 17 Style binding to text-decoration in Angular Remove an item from an array in Angular Remove a component in Angular Delete a component in Angular Use TypeScript enums in Angular templates Set the value of an individual reactive form fields Signal-based components in Angular 17 Angular libraries for Markdown: A comprehensive guide Angular libraries for cookies: A comprehensive guide Build an Angular 14 CRUD Example & Tutorial Angular 9 Components: Input and Output Angular 13 selectors Picture-in-Picture with JavaScript and Angular 10 Jasmine Unit Testing for Angular 12 Angular 9 Tutorial By Example: REST CRUD APIs & HTTP GET Requests with HttpClient Angular 10/9 Elements Tutorial by Example: Building Web Components Angular 10/9 Router Tutorial: Learn Routing & Navigation by Example Angular 10/9 Router CanActivate Guards and UrlTree Parsed Routes Angular 10/9 JWT Authentication Tutorial with Example Style Angular 10/9 Components with CSS and ngStyle/ngClass Directives Upload Images In TypeScript/Node & Angular 9/Ionic 5: Working with Imports, Decorators, Async/Await and FormData Angular 9/Ionic 5 Chat App: Unsubscribe from RxJS Subjects, OnDestroy/OnInit and ChangeDetectorRef Adding UI Guards, Auto-Scrolling, Auth State, Typing Indicators and File Attachments with FileReader to your Angular 9/Ionic 5 Chat App Private Chat Rooms in Angular 9/Ionic 5: Working with TypeScript Strings, Arrays, Promises, and RxJS Behavior/Replay Subjects Building a Chat App with TypeScript/Node.js, Ionic 5/Angular 9 & PubNub/Chatkit Chat Read Cursors with Angular 9/Ionic 5 Chat App: Working with TypeScript Variables/Methods & Textarea Keydown/Focusin Events Add JWT REST API Authentication to Your Node.js/TypeScript Backend with TypeORM and SQLite3 Database Building Chat App Frontend UI with JWT Auth Using Ionic 5/Angular 9 Install Angular 10 CLI with NPM and Create a New Example App with Routing Styling An Angular 10 Example App with Bootstrap 4 Navbar, Jumbotron, Tables, Forms and Cards Integrate Bootstrap 4/jQuery with Angular 10 and Styling the UI With Navbar and Table CSS Classes Angular 10/9 Tutorial and Example: Build your First Angular App Angular 9/8 ngIf Tutorial & Example Angular 10 New Features Create New Angular 9 Workspace and Application: Using Build and Serve Angular 10 Release Date: Angular 10 Will Focus on Ivy Artifacts and Libraries Support HTML5 Download Attribute with TypeScript and Angular 9 Angular 9.1+ Local Direction Query API: getLocaleDirection Example Angular 9.1 displayBlock CLI Component Generator Option by Example Angular 15 Basics Tutorial by Example Angular 9/8 ngFor Directive: Render Arrays with ngFor by Example Responsive Image Breakpoints Example with CDK's BreakpointObserver in Angular 9/8 Angular 9/8 DOM Queries: ViewChild and ViewChildren Example The Angular 9/8 Router: Route Parameters with Snapshot and ParamMap by Example Angular 9/8 Nested Routing and Child Routes by Example Angular 9 Examples: 2 Ways To Display A Component (Selector & Router) Angular 9/8 Tutorial: Http POST to Node/Express.js Example Angular 9/8 Feature and Root Modules by Example Angular 9/8 with PHP: Consuming a RESTful CRUD API with HttpClient and Forms Angular 9/8 with PHP and MySQL Database: REST CRUD Example & Tutorial Unit Testing Angular 9/8 Apps Tutorial with Jasmine & Karma by Example Angular 9 Web Components: Custom Elements & Shadow DOM Angular 9 Renderer2 with Directives Tutorial by Example Build Progressive Web Apps (PWA) with Angular 9/8 Tutorial and Example Angular 9 Internationalization/Localization with ngx-translate Tutorial and Example Create Angular 9 Calendar with ngx-bootstrap datepicker Example and Tutorial Multiple File Upload with Angular 9 FormData and PHP by Example Angular 9/8 Reactive Forms with Validation Tutorial by Example Angular 9/8 Template Forms Tutorial: Example Authentication Form (ngModel/ngForm/ngSubmit) Angular 9/8 JAMStack By Example Angular HttpClient v9/8 — Building a Service for Sending API Calls and Fetching Data Styling An Angular 9/8/7 Example App with Bootstrap 4 Navbar, Jumbotron, Tables, Forms and CardsHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
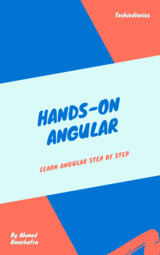