Angular 10/9 SEO: Adding Title and Meta Tags to Your Universal App
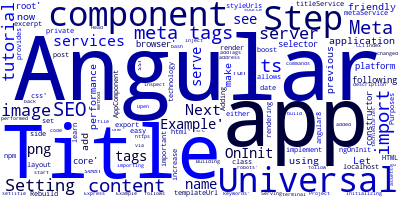
In the previous tutorial, we've seen how to do server-side rendering of our Angular 10 application to make our app SEO friendly and increase the performance. Let's now learn how to add meta tags to our app which is also important for SEO.
Setting Meta and Title Tags in Angular 10 for SEO Purposes
Angular provides the Meta
and Title
services that allows you to either get or set the HTML meta tags and title.
In the previous tutorial, we've performed the following steps:
- Step 1 - Setting up Angular 10 CLI and Initializing a Project
- Step 2 - Setting up Angular 10 Universal
- Step 3 - Building and Serving the App with Express Server
Now, let's do one more step!
Step 4 - Setting Meta Tags and Title
Open the src/app/app.component.ts
file and start by importing the Meta and Title services as follows:
import { Component } from '@angular/core';
import { Title, Meta } from '@angular/platform-browser';
Next, inject both Angular 10 services via the component constructor:
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular 10 Universal Example';
constructor(private titleService: Title, private metaService: Meta) {}
}
Next, import OnInit and implement it:
import { Component, OnInit } from '@angular/core';
import { Title, Meta } from '@angular/platform-browser';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
Next, add the ngOnInit()
method:
ngOnInit() {
this.titleService.setTitle(this.title);
this.metaService.addTags([
{name: 'keywords', content: 'Angular, Universal, Example'},
{name: 'description', content: 'Angular Universal Example'},
{name: 'robots', content: 'index, follow'}
]);
}
Step 5 - Rebuild and Serve your Angular 10 App
Head back to your terminal, rebuild and serve your Angular 10 app using the following commands:
$ npm run build:ssr
$ npm run serve:ssr
If you go to the http://localhost:4000/ address and inspect the source code, you should see the title changed to Angular Universal Example and the meta tags added:
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date: