How to enable CORS in Angular 14
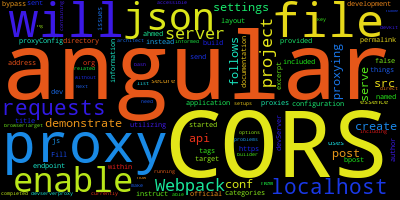
In this post, we will demonstrate how you can enable CORS in Angular 14 by proxying all requests to localhost.
By utilizing the proxies that are provided by Webpack, we can bypass the CORS issues. To get things started, create your Angular 14 project and create a new file in the src/
directory of your project named proxy.conf.json
.
Fill it with the information that follows:
{
"/api": {
"target": "http://localhost:3000",
"secure": false
}
}
This will instruct your development server to proxy all requests that are sent to the /api
endpoint and send them to the localhost:3000
address instead. This file, in essence, uses the settings that is included within Webpack's devServer.proxy
file. The official documentation of their project has a list of all of the settings that are currently accessible.
Next, you will need to make Angular informed of your proxy settings. You can do this by including a proxyConfig
key in your angular.json
file, which will direct Angular to the file containing your proxy configuration as follows:
"architect": {
"serve": {
"builder": "@angular-devkit/build-angular:dev-server",
"options": {
"browserTarget": "<application-name>:build",
"proxyConfig": "src/proxy.conf.json"
}
}
}
Having completed all of the setups, you should now be able to serve your application without running into any CORS-related problems:
ng serve
-
Date: