Generate standalone components in Angular 14
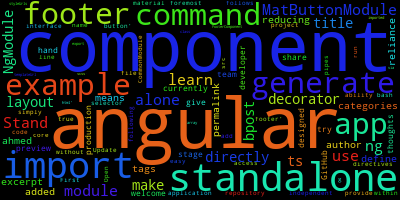
In this example, we'll learn how to generate angular 14 standalone components.
Stand-alone components were added in Angular 14 as a means of reducing reliance on NgModules. Since it is currently in the developer preview stage, you shouldn't use it for any apps that are designed for production.
On the other hand, you are welcome to give it a try and share your thoughts with the Angular team through their GitHub repository.
The command ng generate component <name> --standalone
makes it easy to generate a component that is independent of any module in your application.
First and foremost, stand-alone components provide you the ability to directly add imports (components, directives, pipes, and modules) to the @component
decorator without having to make use of @NgModule
.
Open a command line interface and simply run the following command from within an angular 14 project:
ng g component footer --standalone
You can then access the component's code from the src/app/footer/footer.component.ts
file.
Update the component as follows:
import { Component } from ‘@angular/core’;
import { MatButtonModule } from '@angular/material/button';
@Component({
selector: 'app-footer',
standalone: true,
imports: [
CommonModule,
MatButtonModule
],
templateUrl: './footer.component.html',
styleUrls: ['./footer.component.scss']
})
export class FooterComponent {}
We imported the MatButtonModule
directly via the imports
array of the component's decorator.
-
Date: