Running Node.js in web browsers
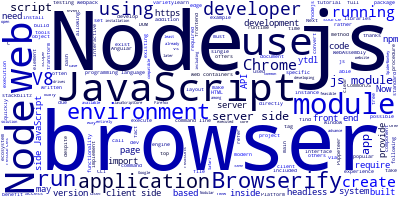
Node.js remains a key expertise for front-end developers due to the vast ecosystem of Node-based tools and applications that are required for modern front-end web development.
For those of you who have primarily written JavaScript that executes in the browser and who would like to gain a better understanding of the server side, Node JavaScript is an excellent method to develop server-side software while also capitalizing on your JavaScript skills.
However, we can also use Node.js to assist with front-end web development. For example, popular client-side libraries such as Angular makes use of CLI or command-line interface tools to enable developers to create projects and even build and deploy them to the cloud.
Learning to build server-side apps using Node is beyond the scope of this scope; rather than that we'll see how to take benefits of running Node modules in the clien's browser.
In this quick tutorial, we'll learn to use and run Node.js modules in the browser then we'll see how it is now possible to run the full Node.js runtime inside the web browser using web containers.
Node.js vs web browsers
The JavaScript programming language is used by both the browser and node.js, although their respective Run Time Environments are distinct.
There are numerous similarities between server-side JavaScript and client-side JavaScript. In addition, there are a number of differences.
There are several significant distinctions between the two, despite the fact that they both use JavaScript as their programming language.
Using the same programming language on both the client and server offers developers a clear edge, since its's difficult to master a new programming language from scratch.
JavaScript is a scripted and interpreted programming language that can be used on both the client and server sides to create interactive web experiences.
It is possible to execute JavaScript on both the browser and Node.js which are both built around a JavaScript engine that provides the execution environment for JS code.
By using JavaScript, you may transform a static web page into an interactive one, enhancing the user experience and providing functionality to web pages.
Node.js apps provide a huge benefit for frontend developers who often use JavaScript: the ability to script both the frontend and backend in a single language.
Making a Node.js app is a very different procedure than building a browser-based application. However, despite the fact that all the code is written in JavaScript, there are many factors that have a substantial impact on the developer's experience.
For instance, the filesystem and server databases are accessible to Node app developers, but in browsers, these resources are restricted and sandboxed for obvious reasons of security.
On browsers, one important aspect is the DOM which refers to Document Object Model and a set of Web Platform APIs such as sessions and cookies.
In a nutshell, the DOM is a tree representation of the web page document. It contains the objects/nodes that refer to the layout elements and contents of a web page. It's also a interface that provides the JavaScript methods that you can call to create create and remove new elements and traverse the tree to find any element on the page using CSS selectors such as IDs and classes.
On the other hand, Node.js doesn't have the DOM and developers are unable to access the document, window, and other browser objects.
There are instances on the web browsers when you are forced to use an earlier version of JavaScript since the standard advances so quickly yet browsers are sluggish to update.
Before delivering your code to the browser, you can use Babel to convert it to ES5 compatibility, but in Node.js, you won't need to.
Node.js supports both the CommonJS and the modern EcmaScript module systems, whereas browsers are just beginning to implement the ES Modules standard.
This implies that in Node.js, you can use both require()
and import, however in the browser, you can only use import.
In addition, Node.js gives you total control over your environment, allowing you to choose the version of Node.js your application will run on as a developer.
When comparing this to a browser, the environment is determined by the version of the browser being used by end users so as a front-end developer, you must pay care when developing your app since features may not exist on specific browsers, causing your app to crash.
Node.js vs Chrome
Both Node.js and Chrome are built on top of v8, and many of the Node.js libraries can be already executed in Chrome.
The JavaScript engine that drives Google Chrome is called V8. It is the component that runs our JavaScript when surfing using Chrome.
Recently Microsoft built Edge a new and modern browser that is also built on top of V8.
Other popular browsers such as Firefox is based on SpiderMonkey and Safari on JavaScriptCore.
V8 is Google's open source JavaScript and WebAssembly engine built on C++. It is used in a variety of apps, including Chrome and Node.js. It is compatible with Windows 7 or later, macOS 10.12 or later, and Linux systems powered by x64, IA-32, ARM, or MIPS CPUs. V8 may be utilized independently of any C++ program or as an integrated component in any C++ application.
V8 offers the JavaScript runtime environment. The browser provides the DOM and other Web Platform APIs.
JavaScript as a programming language is entirely agnostic of the browser or environment in which it is executed.
V8 was adopted to run Node.js in 2009, and as Node.js's popularity grew, V8 became the engine that currently drives an astounding amount of server-side JavaScript code.
The Node.js ecosystem is massive, in part because to V8, which powers desktop applications via projects like Electron.
You can check which version of V8 engine is included with your Node.js installation using the following command:
node -p process.versions.v8
Why running Node modules in the browser?
Node.js provides the world's largest repository of open source modules, which tackle a wide range of common and unique development issues. You can quickly find a module for practically any functionality you require, allowing you to focus on addressing your specific requirements rather than reinventing the wheel and increasing your productivity.
You can use these modules if you solely work in Node.js environments, such as while developing a server-side JavaScript application. What if you need to create a client-side JavaScript application? Is it possible to run Node.js in a browser? To put it succinctly, no, at least not directly!
Can Node.js run in a browser
As a front-end JavaScript developer, you could need to run Node.js code in the browser for a variety of reasons, including: - You find a handy Node.js-based npm package that solve a problem you are working on! - You would like to leverage Node.js APIs suhc as crypto - You prefer to write modules in the CommonJS approach and so on.
The Node.js runtime does not exist on the client; all you have is a browser that cannot use Node.js modules. What a colossal waste, we hear you exclaim!
However, guess what? Thanks to some creative engineers, it is now feasible to use Node.js modules in browsers, but not directly.
Being able to call Node.js modules from JavaScript running in the browser has many advantages because it allows you to use Node.js modules for client-side JavaScript applications without having to use a server with Node.js just to implement functionality that is already available as a Node.js module that is available via an npm package.
Existing ways for running Node modules inside the browser
It is possible to meet at least some of the aforementioned objectives by using existing tools such as: - Webpack.js, - Browserify, - And Nodular.js, among others.
For each solution, a source code transformation is required, either in advance (in the case of webpack and browserify) or in real time in the case of nodular. They may be appropriate for your purposes, but it's worth noting that they are just attempts to reproduce or emulate specific behaviours inside the browser.
Introducing Browserify
The official website describes Browserify with the following sentence:
Browserify lets you require('modules') in the browser by bundling up all of your dependencies.
In order to use the modular system of Node.js to structure your JavaScript applications on the client side, you can use Browserify to require JavaScript modules from JavaScript running on the browser.
Browsers lack the Node.js platform's require/export and module import systems; therefore, Browserify was developed to make it possible to import Node.js modules.
In order to use Browserify, you must transform your Node.js scripts into a regular javascript file that can be included in your website's HTML script element or through any other browser mechanism.
Suppose you have written your application source code using require syntax to import a useful Node.js module. Now to be able to use your application in the browser with no error you need to use Browserify and run the following command:
browserify app.js -o bundle.js
Browserify resolves all dependencies, concatenates them, and bundles them into a single JavaScript file that can be included with a single script tag.
You can also use browserify to take advantage of Node.js' modular and import system, i.e. require module.exports, to structure your client side JavaScript applications, particularly if you are familiar with this system, so you don't have to learn another importing system for the browser, such as requirejs or commonjs.
How To use Browserify?
To install browserify, first enter your command line terminal and type the legendary npm install
command as follows:
npm install -g browserify
Next create your example project folder
mkdir ytdl
Create a main.js
JavaScript file inside ytdl
Next you should install the module you want to use or to convert to browser compatible script
npm install -g ytdl-core
Next in main.js
require the module as you do normally with node.js modules:
var yt = require('ytdl-core');
console.log(yt);
Now let the magic begins, call browserify with the input is main.js
and give it a name for the output
browserify main.js -o ytdl.js
...
Now you can use your script as easily as using a script tag
<script src="ytdl.js"></script>
...
Replicating Node environments in browsers
Now that, we've seen how to compile a run a single Node module in the browsers, another question that arises is that can we repliate a full node environment?
Many solutions exist that tried to replicate the Node.js environment inside the browser.
Can we, for example, build the webpack code in the browser rather than the server's nodejs environment? The benefit of this is that we no longer need the server's capabilities for compilation.
This issue may be solved in a variety of ways. For instance, codesandbox, which is described as a browser-based compilation technique, may be used to package react, vue, and other browser-based libraries. It is also clear that codeandbox provides a browser version of webpack.
Running a headless browser within Node
We've seen ways to run Node in web browsers but how about the inverse, can you run a web browser with Node.js? In fact, you can thanks to headless browsers and Node libraries such as Playwright for running Chrome, Firefox and Safari or Puppeteer for running headless Chrome browser and others.
Puppeteer is a Node module that exposes a high-level API for administering Chrome or Chromium through the DevTools Protocol. Puppeteer is set to run full (non-headless) Chrome or Chromium by default.
If a web browser does not have a graphical user interface, it is said to be headless. In an environment comparable to common web browsers, headless browsers automate the administration of a web page, but they use a command-line interface instead or a Node.js script. Aside from the fact that they can display and interpret HTML the same way a browser would, they are especially beneficial when it comes to testing web applications since they are able to include style components like page layout, color, font selection and the execution of JavaScript and Ajax.
The majority of tasks that may be accomplished manually in the browser can be accomplished using headless browsers and Node.js, for example:
- Create page screenshots and PDFs.
- Crawl an SPA (Single-Page Application) and create pre-rendered content (sometimes referred to as "SSR" (Server-Side Rendering)).
- Automate form submission, user interface testing, and keyboard input, among other tasks.
- Create an automated testing environment that is up to date. Conduct your tests directly in Chrome, using the most recent JavaScript and browser capabilities.
Running Node.js in browsers with web containers
As a front-end web developers, you may not need to use Node.js to build apps since the front-end doesn't and can't have access ro server resources directly but often via a REST or GraphQL API.
However, nowadays modern web development frameworks and libraries such as Angular, React or Vue provides a set CLI tools that are built on top of Node.js.
This means that Node is required on the development of front-end apps even if you don't target or develop for the server side.
A few years ago, it seemed clear that the web was nearing a turning point. In light of the release of WebAssembly and new powerful APIs, it seems feasible to develop a WebAssembly-based runtime robust enough to run Node.js entirely inside a web browser. The goal was to provide a development environment that was faster, more secure, and more consistent than local development environments.
As a consequence, the stackblitz team, announced the development of web containers. Web containers make it easy to build a fully functional Node.js environment that boots in milliseconds, is online, and can be shared with others. VS Code's rich editing experience, a complete terminal, npm, and more are loaded into the environment.
Tht means that you can run the Node.js runtime natively, inside the browser, for the first time in the history of web development.
You can try that at StackBlitz.com.
Conclusion
A Node.js module running on a browser is incredibly advantageous and helpful for developers. We learned how to run our Node module in a browser in this tutorial. Due to NodeJS's reliance on server-side technologies, it is impossible for developers to run its modules in their browsers. As a result, Browserify was developed. It's easier to make Node modules browser-friendly thanks to its simple installation procedures and easier for JavaScript developers to take adavnatge of the existing Node modules to create client-side applications.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date: