How to Open URLs in Browser with Ionic 5/Angular,Cordova InAppBrowser plugin and Ionic Native
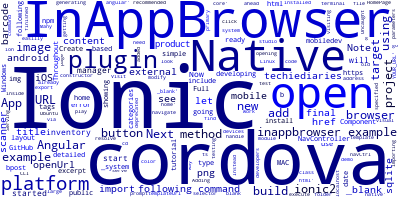
Throughout this tutorial, we are going to see a detailed example showing how to open external URLs in Ionic 5 mobile apps based on Angular using the Cordova plugin InAppBrowser and Ionic Native.
SEE ALSO:
Full Ionic 5/Angular Mobile App with Ionic Native and InAppBrowser
AND
Ionic 2/3 : Using Cordova SQLite and Barcode Scanner plugins to build a product inventory manager
Now let's get started!
Create a New Ionic 5/Angular Project
We will start by generating a new Ionic 5/Angular project with Ionic CLI 4 so open up your terminal if your are developing under a Linux/MAC system or your command prompt under Windows and type!
ionic start ionic-inappbrowser-example blank --type=angular
Note: Under Windows the recommended way to work with Ionic 5 is through visual studio.
Next, navigate inside your project folder:
cd ionic-inappbrowser-example
Add your target platform using the following command:
cordova platform add android
We are developing with Ubuntu so we can only target Android devices. If you are under a MAC you can target iOS too using:
cordova platform add iOS
Note : If you don't have Cordova already installed you can easilly install it via npm with
npm install -g cordova
Adding the InAppBrowser Cordova Plugin
Next, you just need to add the InAppBrowser plugin using the following command:
cordova plugin add cordova-plugin-inappbrowser
Now we are ready to add some code to our project to open external URLs. So go ahead and open the home.ts
file
and modify it to look like the following:
import { Component } from '@angular/core';
import { NavController , Platform} from 'ionic-angular';
import { InAppBrowser } from 'ionic-native';
@Component({
selector: 'page-home',
templateUrl: 'home.html'
})
export class HomePage {
constructor( public platform: Platform,public navCtrl: NavController) {
}
openUrl() {
this.platform.ready().then(() => {
let browser = new InAppBrowser("https://www.techiediaries.com",'_blank');
});
}
}
We have started by importing the InAppBrowser plugin from the ionic-native
module then we have
added the openUrl()
method that will handle opening the specified URL.
Next open your your template and add the button to test the method:
Note: Don't use the old
.open()
method
InAppBrowser.open(url, "_system");
Many developers are getting this error
Uncaught Error: Can't resolve all parameters for InAppBrowser: (?, ?, ?).
Because it is deprecated with Ionic Native. Instead you should use the following method:
let browser = new InAppBrowser('YOUR_URL', "_system");
Serving your Ionic 5 App
Next, just execute the following command:
ionic serve
You can the play with your demo on the browser by visiting the http://localhost:8100/
address.
When you are finished testing, you can build your app for you target platform using the following command:
ionic build android | iOS
You can find the example application we have build in this GitHub repository{target:_blank}
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Ionic 5/Angular and Cordova SQLite Storage Example Boosting the Performance of Ionic 5 Apps with Crosswalk Printing with Ionic 5, Ionic Native 5 and Cordova checking Ionic 5 Platform: Device Information Building Augmented Reality Apps with Ionic 5/4 and Wikitude Cordova Plugin Use Geolocation in Ionic 5/Angular Apps with Ionic Native 5 Using Camera with Ionic 5 and Ionic Native 5 Ionic 5 Cloud Ionic Native for Ionic 5 with Camera Example How to Open URLs in Browser with Ionic 5/Angular,Cordova InAppBrowser plugin and Ionic Native How to Update Ionic Native Version 5