Reset file input with React
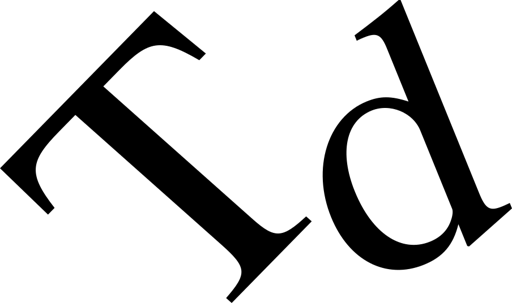
In React, when we provide a reference to a ref
prop in an HTML element:
<input ref={aRef} />,
React sets the .current
property of the ref variable to the corresponding DOM node of the HTML element.
This means, we can access all the DOM properties of the element via the aRef.current
object. Among them, the value object.
This also means that we can reset the value by passing null to value:
aRef.current.value = null;
In a nutshell, you can assign a ref to the file input, which enables you to reset its value.
Here is a full example of how to reset file input in React.
import * as React from 'react';
import { useRef } from 'react';
import './style.css';
export default function App() {
const aRef = useRef(null);
const handleFileChange = event => {
const fileObj = event.target.files && event.target.files[0];
if (!fileObj) {
return;
}
console.log('fileObj: ', fileObj);
};
const resetInput = () => {
aRef.current.value = null;
};
return (
<div>
<h1>How to reset file input in React!</h1>
<input ref={aRef} type="file" onChange={handleFileChange} />
<button onClick={resetInput}>Reset </button>
</div>
);
}
First, we use the useRef
hook define a reference then we define two functions for handling file change and resetting the file input value.
We bind the resetInput function to the onClick event of a button that will reset the input file value when clicked!
The resetInput value simply assings null to the current.value
object.
You can also run the example from stackblitz
-
Date: