Build REST API with Laravel 9
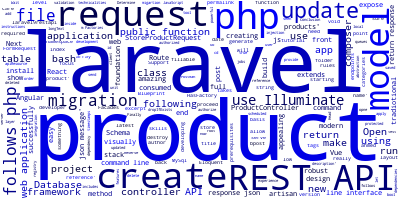
In this post, we'll show you how to build a REST API with laravel 9. Before you can proceed with this tutorial, you need to install the latest version of laravel, and make sure you have the following prerequisites:
- PHP 8.0.2+
- MySql
- Composer
Laravel 9 is a full-stack web application framework with a robust and visually appealing design that is easy to use to create tradiotional web applications and modern web applications that expose REST APIs that could be consumed by front-end applications such as Angular, React or Vue.js.
Using a web framework as a basis and starting point for developing your application will allow you to focus on creating something really amazing while the foundation takes care of the low-level technicalities for you.
You can also use laravel 9 to build a REST API that can be consumed by a JavaScript client application.
Create a laravel 9 project
You can create a laravel 9 by creating using composer as in the following instructions.
Open a new command-line interface and and run the following command:
composer create-project laravel/laravel laravel9restapi
Wait for composer to create the project's files and install the dependencies from its registry then run the following commands to serve your laravel 9 application using a local development server:
cd laravel9restapi
php artisan serve
Create a new laravel 9 model
Open a new command line interface and run the following command from the project root folder to generate a product model with a migration file:
php artisan make:model Product -m
Next, opne the migartion file inside the database/migrations
folder and update it as follows:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('products', function (Blueprint $table) {
$table->id();
$table->string('reference');
$table->string('name');
$table->longText('description');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('products');
}
};
Following that, open the app/models/Product.php
file and update the fillable array as follows:
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Product extends Model
{
use HasFactory;
protected $fillable = ['reference', 'name'];
}
Create the REST API controller
After creating the model and migration file for products, let's now create the controller. Head back to your command-line interface and run the following command:
php artisan make:controller Api\\ProductController --model=Product
Next, open the app/Http/Controllers/Api/ProductController.php
file and update the index()
function it as follows:
public function index()
{
$products = Product::all();
return response()->json([
'products' => $products
]);
}
Next, update the store()
method as follows:
public function store(StoreProductRequest $request)
{
$product = Product::create($request->all());
return response()->json([
'message' => "Product saved successfully!",
'product' => $product
], 200);
}
Next, update the update()
method as follows:
public function update(StoreProductRequest $request, Product $product)
{
$product->update($request->all());
return response()->json([
'message' => "Product updated successfully!",
'product' => $product
], 200);
}
Finally, update the destroy()
method of the controller class as follows:
public function destroy(Product $product)
{
$product->delete();
return response()->json([
'message' => "Product deleted successfully!",
], 200);
}
Go back to your command line interface and , generate a request as follows:
php artisan make:request StoreProductRequest
Open the app/Http/Requests/StoreProductRequest.php
file as follows:
<?php
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
class StoreProductRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*
* @return bool
*/
public function authorize()
{
return true;
}
/**
* Get the validation rules that apply to the request.
*
* @return array
*/
public function rules()
{
return [
"name" => "required",
"reference" => "required"
];
}
}
Create the REST API endpoints
After that, open the routes/api.php
file and add the REST API endpoints as follows:
<?php
use App\Http\Controllers\Api\ProductController;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
Route::apiResource('products', ProductController::class);
You can test these API routes using a tool like Postman to send API requests to the laravel 9 application.
Conclusion
We've seen how to install laravel 9 by creating a new project using composer. In order to provide an amazing developer experience, Laravel includes features like robust dependency injection, a powerful database abstraction layer, queues and scheduled jobs, unit and integration testing, and more.
Laravel is a framework that can adapt with your skills, whether you are beginner to PHP web frameworks or have years of expertise. Laravel 9 assists you in taking your initial steps as a web developer or give you a push as you advance your skills.
-
Date: