Fix CORS in Angular 13 App with CLI Proxy Configuration
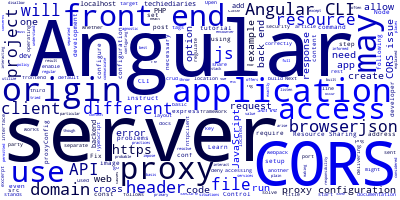
In this article, we'll go through how to use the Angular CLI proxy setup to address CORS errors in our front-end Angular 13 project.
CORS, which stands for Cross-Origin Resource Sharing, enables a server to regulate which front-end JavaScript clients may access server resources. It works by delivering HTTP headers with HTTP responses that instruct web browsers whether to allow or deny frontend JavaScript code from accessing responses.
CORS headers are basic HTTP headers that instruct a browser to enable a web application operating at one origin (domain) to access particular resources from another origin's server.
Browser security disallow you from making cross-domain requests except if the HTTP response has a Control-Allow-Origin
header with a *
value or the domain of your client.
CORS issues are framework-agnostic and may occur in any front-end JavaScript application built with plain JS, React or Vue.js, etc. but in our case, we'll see how to solve CORS issues in the context of an Angular 13 application.
What causes CORS errors in Angular development
A front-end developer's primary responsibility is to access back-end APIs. It is also probable that your front-end application may need to communicate with a server from a different location on a regular basis. For example, you might use your own server, or you could use a third-party API that your application needs.
You may encounter a cross-origin resource sharing (CORS) issue in any of these situations. What exactly is CORS? What are the best practices for interacting with your back-end services? How to utilize CORS in an Angular application will be explained in this post.
Because of security concerns, web browsers impose a "same origin" policy on all web applications. Browser clients are only permitted to interact with servers that share their origin. In the case of Angular, we often need to create a full-stack application with separated client and server apps. As a result, if your front end tried to access a resource from your server that is located in a location with different origins, the browser would throw an error.
Your browser must be informed that your client and server may share resources even if they are located in different places by configuring cross-origin resource sharing (CORS).
On development; since Angular CLI offers a development server that runs on localhost:4200
by default, if you use a back-end server that runs on a different domain, you may have CORS issues if your server is not correctly setup.
Even though your backend runs on localhost, it will listen on a different port, which is considered as a separate domain.
How to resolve CORS issues with Angular 13
We now understand why CORS problems may arise while implementing your Angular application. How can they be fixed?
There are two options:
- Configure the server to transmit the necessary CORS headers
- Set up the Angular CLI proxy
The apparent approach is to correctly setup the backend server, however this is not always achievable. You may not have access to the server code, for example.
Also check out an example of how to configure CORS with TypeScript and Node/Nest.js backend
Most server languages and frameworks provide easy APIs to configure CORS. For example, in PHP, you can simply add the following lines to your PHP file:
header('Access-Control-Allow-Origin: *');
In Node.js and Express server, you can use the cors
package (npm i cors --save
) as follows:
const cors = require('cors');
const express = require('express');
const app = express();app.use(cors());
In this angular tutorial, we will learn step by step how to use Angular CLI proxy to fix CORS issues.
Prepare your Angular 13 project
At this step, we expect that you alreay have an Angular project with some code to send HTTP requests and CORS. Otherwise, you need to create a project and some code for sending requests to a server.
Open a new command-line interface and navigate to the root folder of your project.
Create a proxy configuration file for Angular 13 CLI
Next, create src/proxy.conf.json
file and add the following content:
{
"/api/*": {
"target": "http://localhost:3000",
"secure": false,
"logLevel": "debug"
}
}
This configuration file specifies that any HTTP request which starts with the /app/
path will be sent to the proxy which will redirect it to the target
hostname.
The secure
option is used to enforce usage of SSL.
See all the available options from webpack dev server documentation.
Add a proxyConfig
key to angular.json
Next, open the angular.json
file and add a proxyConfig
key under the serve->options
that points to the src/proxy.conf.json
file as follows:
"architect": {
"serve": {
"builder": "@angular-devkit/build-angular:dev-server",
"options": {
"browserTarget": "your-application-name:build",
"proxyConfig": "src/proxy.conf.json"
},
Serving your Angular 13 application
Finally, use the following command to start the dev server with this proxy configuration:
$ ng serve
If you do any changes in the proxy configuration file afterwards, make sure to re-run the ng serve
command.
You can find more information about the proxy configuration in the Angular CLI from the docs
Conclusion
As a re-wrap, when developing with Angular 13, developers may encouter CORS errors.
Accessing back-end APIs is one of the most important tasks of a front-end developer. Furthermore, your front-end application will frequently need to connect with a server of a different origin. This might be your own server or a third-party application programming interface that your application requires.
In any of these scenarios, it's possible that you'll run into CORS problems if you use Angular CLI with a back-end server running on a different domain than the one provided by the CLI by default (localhost:4200
).
A separate port is used to represent a different domain even if your backend server is operating on your local machine's IP address.
In this post, we'll went over how to leverage the Angular CLI proxy configuration to solve CORS problems in our front-end Angular 13 project.
Servers may control which JavaScript clients can access server resources using CORS (Cross-Origin Resource Sharing). Front-end JavaScript code may access responses only if it is allowed or denied by HTTP headers that are sent with the response to an HTTP request.
HTTP headers designed for CORS instruct a browser to allow a web application from one origin (domain) to access resources from another origin's server.
-
Date: