Angular 13 selectors
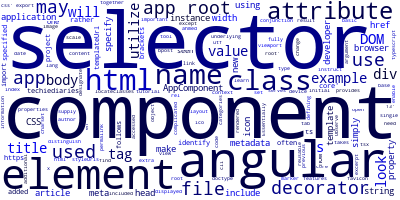
Angular 13 provides the core @Component
decorator to make components out of typescript classes. This decorator takes an object as an argument, which includes a set of attributes. In Angular, a decorator is used to designate a class as a component and to supply additional metadata defining which features may be accessed by the underlying component.
An Angular decorator is a class that serves as a marker for a component and provides extra information about the underlying component's capabilities, such as which features are accessible.
The component’s decorator accepts attributes as metadata, and the object comprises key-value pairs such as selector, style, or styleUrl. All of these attributes may be used together to create a from component’s class a fully reusable piece of code for controlling a certain area of the Angular application. In this article, we’ll look specifically at the selector attribute with examples.
In a nutshell, the selector property enables angular developers to specify how the component should be included in html templates.
It instructs Angular to build and insert an instance of the component wherever the selector tag is found in the parent html file of your angular application.
The selector receives a string value that specifies the CSS selector that will be used by Angular to identify the element in the DOM. We'll utilize it in html templates to include components. If you look at the index.html
file in your angular project, you should find that the body has an <app-root>/app-root>
tag.
We're going to start a new angular project using angular cli. The new project is generated with a component, called AppComponent, which contains an app-root
selector as will be seen next.
What is an angular selector?
We turn a typescript class into an angular component using a decorator with a bunch of metadata attributes. A selector is one of these attributes that we often employ in conjunction with the other component configuration metadata to make a fully working and reusable component.
A selector is often used to identify individual components and to determine how the particular component is rendered in the actual DOM. It’s similar to how we use standard HTML tags to include and select DOM elements except that the angular selector is only available in the context of the application or the module where the component is declared.
Component selectors are CSS selectors that specify how Angular locates a given component inside a Html document. While we often use element selectors (<app-root>/app-root>
), this may be any CSS selector, from a CSS class to an attribute.
When we use the Angular CLI 13 to generate a new component, the newly formed component looks as follows:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
title = 'Hello Angular 13!';
}
Observe that in app.component.ts
, we have one property named selector, as well as the distinctive name used to distinguish the app component in the DOM after it is displayed in the browser. The selector app-root
would result in the component being referenced in the HTML code as <app-root>/app-root>
.
After running our angular 13 app in the browser, we can open the browser developer tools and go to the Elements tab, where we can view the rendered component.
As you can observe, the <app-root>
element is displayed first since it is the root component of our application. If there are any descendant components, they are all placed within the root selector.
Essentially, the component's selector attribute is simply a string that is used to identify the component’s element in the DOM.
The selector name may include an app as a prefix by default when the component is created, but we may change that. Remember that two or more component selectors cannot be the same.
Let's have a look at how we may utilize the selector in Angular 13.
Selectors are also important for preserving uniformity in the names of component selectors and custom specs.
Selectors can be used as an element’s name or attribute.
While it is suggested to utilize element selectors when introducing new components (like we did with app-root
), theoretically any other selector may be used.
You may make the selector as basic or as complicated as you would like, but as a rule of thumb, avoid complicated element selectors except if you possess a very reasonable justification not to.
The basic component requires essentially a selector (to instruct Angular 13 on how to locate the component's instances) and a template (which Angular 13 should render when it finds the element). The component decorator's remaining properties are mandatory. We specified in the earlier example that the AppComponent
will be loaded when Angular 13 encounters the app-root
selector and that the app.component.html
file would be shown when Angular 13 identifies the element.
For instance, below are many approaches for specifying the selector property and using it in HTML.
- name: Use element name as selector.
- .class: Use a class name as a selector.
- [attribute]: Use the name of the attribute as a selector.
We've seen an example of using a selector as an element name, but we're not restricted to that. A selector may also be used as an attribute of an element, just like any other HTML element.
Just like the properties associated with HTML elements such as name, id, class, href, src, value, and others; a selector may also be used as an attribute in Angular 13.
This is rather simple to manage if we need to experiment with component input coming directly from the HTML control/element.
We may use brackets []
in conjunction with a selector, as seen below:
import { Component } from '@angular/core';
@Component({
selector: '[app-root]',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
name = 'Angular 13';
}
We've simply added an extra brackets to the selector value [app-root]
, thus we must now utilize the selector as an attribute rather than an element.
Open the index.html
file and change the <app-root>
markup to look like this:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>My Angular 13 app</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<div app-root> </div>
</body>
</html>
As you tell from the screenshot, the <div>
tag, together with the selector, now works as an element. This indicates that the component selector we specified behaves as an attribute rather than an element.
That's how we distinguish a selector as an attribute from a selector as an element by simply adding []
brackets to the selector string.
Angular 13 selector as a class
Already in this tutorial, we've used a selector as an element and an attribute, however we may additionally utilize it as a class.
We simply need to specify the selector value as the class, and the element will be changed to the class in the HTML DOM, exactly as we did in the previous cases.
Update the app.component.ts
file as follows:
import { Component } from '@angular/core';
@Component({
selector: '.app-root',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
name = 'Angular 13';
}
We simply added a single dot operator, .
before the selector name, so we can use selector as a class name as follows:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>My Angular 13 app</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<div class = "app-root"> </div>
</body>
</html>
To define the class name in this html file, we should use the class property, however as you can see, we have utilized the selector name as a class value.
We can now view the results in the Elements tab of the browser developer tool.
Along with the <div>
element, the class name, app-root
, which is the selector name that we specified in the component file, is added.
We can still display the child elements into the component if we set the selector as a class, like we did in the previous example.
Conclusion
In this article, we learnt about the Angular 13 selectors and how to apply them in various contexts. The selector is one of the important attributes that represents a single component.
-
Date: