Angular 13 app root component
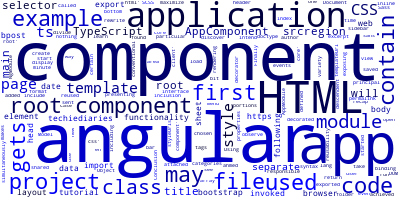
In an angular 13 application, a component is responsible for presenting a certain region of the user interface. For example, a component may be used to create the main page of your application, and if you intend to maximize code reusability even more, you can further divide down the main page into separate components, such as the menu bar, principal area, and bottom regions.
The header and sidebar components of your application may be reused on other pages of your application sans having to rewrite the same code for the elements that are shared by all, or just some, portions of your application.
What is the root component of Angular project
The root component of Angular project is the first component that gets called when you start the application.
Angular components are nothing more than a TypeScript class decorated with the @Component
decorator, which is a functionality that is exported from the angular 13 core module; and an HTML template, which may be used inline or saved as a separate HTML file. The HTML template makes use of particular angular template syntax to display data while simultaneously binding properties and events from the component's class.
A component may be attached with one or more style sheet files that contain styles for the view in the format you've chosen for your project, such as CSS, SCSS, or Sass.
If you take a minute to return to your application for a bit. In the src/app
folder, you'll discover a variety of files that include TypeScript code, an HTML template, and a CSS style sheet for the app component, including the following:
- The
app.component.css
file that contains the CSS styles for the component - The
app.component.html
file that contains the HTML code for rendering the component - The
app.component.ts
file that contains the TypeScript code for exposing the component data and functionality.
When the app is run for the first time, the root component is what gets first invoked. This is achieved by the inclusion of the component in the bootstrap array of the application module.
For example, the following code is found in the src/app/app.module.ts
file that contains the application module:
// [...]
import { AppComponent } from './app.component';
@NgModule({
// [...]
bootstrap: [AppComponent]
})
export class AppModule { }
The root component is also added in the src/index.html
file of Angular 13 project, which is the file that gets sent when you open your Angular 13 application from a web browser:
<!doctype html>
<html lang="en">
<head>
<!-- [...] -->
</head>
<body>
<app-root></app-root>
</body>
</html>
Using its selector, you can observe that the root component is being used in the same way that a conventional HTML element would be used. In this case, Angular will load the component and all of its descendent components into the Document Object Model (DOM).
Finally, this is how the root component of a new Angular 13 project is defined:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'client';
}
You can see more examples from this angular tutorial.
Conclusion
As a recap, the root component of angular project is the first component that gets invoked when the application is started in the web browser.
-
Date: