Angular 17: Enhanced Control Flow Simplified with Examples

Angular 17 introduces a revamped built-in syntax for handling control flow within templates. This updated syntax is designed to be more declarative, making it both easier to comprehend and simpler to implement compared to the previous method involving directives like NgIf and NgFor.
Let's delve into some examples of how to employ this new control flow syntax effectively in Angular 17:
Example 1: If Statement
@if (isLoggedIn) {
<span>Welcome, </span>
} else {
<a href="/login">Login</a>
}
In this scenario, the @if block will render the enclosed content only if the condition is true. Otherwise, the @else block will be rendered.
Example 2: Else If Statement
@if (user.role === 'admin') {
<span>Admin dashboard</span>
} @else if (user.role === 'editor') {
<span>Editor dashboard</span>
} @else {
<span>User dashboard</span>
}
The @else if block allows for specifying multiple conditions. It renders the content within the first matching @else if block. If none match, the @else block is used.
Example 3: For Loop
<ul>
@for (let item of items) {
<li></li>
}
</ul>
The @for block iterates through the content within it for each item in the iterable object.
Example 4: Empty Block
<ul>
@for (let item of items) {
<li></li>
} @empty {
<li>No items to display</li>
}
</ul>
The @empty block is rendered if the iterable object is empty.
Example 5: Context Properties
<ul>
@for (let item of items; track item; let i = $index) {
<li>. </li>
}
</ul>
The @for block offers context properties like $index to access the current item's index and other relevant information.
Example 6: Deferral
<ul>
@defer {
@for (let item of items) {
<li></li>
}
}
</ul>
The @defer directive postpones rendering a block until specified conditions are met. For instance, it can delay rendering until a data fetch operation completes.
Benefits of the New Control Flow Syntax
The upgraded control flow syntax in Angular 17 brings several advantages:
- Enhanced declarative readability and simplicity.
- Improved efficiency compared to previous directive-based approaches.
- Support for deferral, potentially boosting performance.
Conclusion
The fresh control flow syntax in Angular 17 represents a substantial improvement over prior methods involving directives. It offers enhanced declarative power, efficiency gains, and the ability to defer rendering. I encourage you to explore and integrate this new syntax into your Angular applications for a more streamlined development experience.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
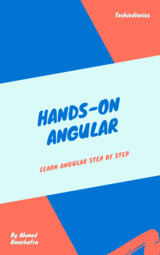