A Step-by-Step Guide to Using RxJS combineLatestWith

RxJS is a powerful library for handling asynchronous operations in JavaScript applications. One of its operators, combineLatestWith, enables you to combine the latest values from multiple observables into a single observable. In this step-by-step guide, we'll explore how to use combineLatestWith effectively in your RxJS code.
Prerequisites: Before you begin, make sure you have the following prerequisites:
- Basic knowledge of JavaScript and RxJS.
- Node.js installed on your system.
- A code editor (e.g., Visual Studio Code).
Step 1: Set Up Your Environment
Start by creating a new JavaScript or TypeScript project and setting up the necessary dependencies. You can use npm or yarn to manage your project.
# Create a new project folder
mkdir combineLatestExample
cd combineLatestExample
# Initialize your project (choose one)
npm init -y
# OR
yarn init -y
# Install RxJS
npm install rxjs
# OR
yarn add rxjs
Step 2: Create Your First Observables
In this example, let's imagine you have two observables, observable1 and observable2, which emit values over time. We'll create simple observables for demonstration purposes:
// Import RxJS
const { of, interval } = require('rxjs');
const { combineLatestWith } = require('rxjs/operators');
// Create observables
const observable1 = of('A', 'B', 'C');
const observable2 = interval(1000); // Emits values every second
Step 3: Use combineLatestWith Operator
Now, let's combine the latest values from observable1 and observable2 using the combineLatestWith operator. This will create a new observable that emits an array containing the latest values from both observables whenever either of them emits a new value.
// Combine observables using combineLatestWith
const combinedObservable = observable1.pipe(
combineLatestWith(observable2)
);
Step 4: Subscribe and Output the Results
To see the combined values in action, subscribe to the combinedObservable and log the results.
// Subscribe to the combined observable
combinedObservable.subscribe(([value1, value2]) => {
console.log(`Latest values: ${value1} from observable1, ${value2} from observable2`);
});
Step 5: Run Your Code
Save your code in a file (e.g., main.js), and then run it using Node.js:
node main.js
You should see the combined values being logged to the console as they change over time.
Conclusion:
In this step-by-step guide, you've learned how to use the RxJS combineLatestWith operator to combine the latest values from multiple observables. This operator is valuable for scenarios where you need to react to changes in multiple data sources simultaneously.
Feel free to adapt this example to your specific use cases and explore more advanced features of RxJS as you continue to build powerful and reactive applications.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
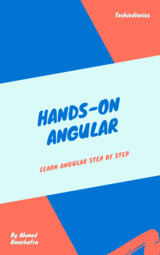