Angular 16 Injecting Service without Constructor

In Angular 14+, there is a technique to inject a service without utilizing a constructor. This can be achieved by making use of the inject() function. The inject() function allows you to retrieve a service instance based on its injection token from the currently active injector. By utilizing this method, you can inject services into your components, directives, pipes, or modules without the need for constructor injection.
Here's a detailed guide on how to use the inject() function to inject a service:
Injecting a Service Using inject()
:
Import the inject() function: Start by importing the inject() function from the '@angular/core' package in your TypeScript file where you intend to perform the injection.
import { inject } from '@angular/core';
Inject the Service: To inject the service using inject(), you simply pass the type of the service as a parameter to the function. This retrieves the service instance associated with that type from the injector. For example:
export class MyComponent {
private service: MyService;
constructor() {
this.service = inject(MyService);
}
}
In this example, the MyService is injected into MyComponent without the need for a constructor.
Access the Injected Service: Once the service has been injected using inject(), you can access it like any other property of the component. You can call its methods or access its properties as needed, just like you would with constructor injection:
this.service.doSomething();
Using inject() with Other Types of Classes:
It's important to note that you can use the inject() function to inject services into various types of classes, not just components. You can use it with directives, pipes, modules, or any other Angular construct. Here's an example of injecting a service into a directive:
import { Directive, inject } from '@angular/core';
@Directive({
selector: '[myDirective]',
})
export class MyDirective {
private service: MyService;
constructor() {
this.service = inject(MyService);
}
// ...
}
Use Cases for Injecting Services Without Constructors:
There are specific scenarios where injecting a service without using a constructor can be advantageous:
Avoiding Circular Dependencies: When two services have mutual dependencies, direct constructor injection can result in circular dependencies. Injecting services without constructors can help circumvent this issue.
Deferring Injection: There might be situations where you need to delay the injection of a service until a certain point in a component's lifecycle. For example, you may want to inject the service based on the value of an input property, which can be more easily achieved without constructors.
Injecting Services into Non-Component Classes: While Angular components are the most common recipients of service injections, you can also inject services into other types of Angular classes, such as directives, pipes, or modules, using this technique.
Best Practices:
Injecting services without constructors can make your code less intuitive and more challenging to maintain. Therefore, it's advisable to exercise caution and use this technique judiciously. Here are some best practices to consider:
Use Sparingly: Only resort to injecting services without constructors when there's a clear and compelling need to do so. Reserve it for scenarios where constructor injection is not feasible or causes issues.
Avoid Reusable Components: It's generally not recommended to use this technique with components that are designed for broad reuse. Constructor injection tends to be more transparent in such cases.
Consider Testability: Be mindful of how this approach affects the testability of your classes. It may be more challenging to test components that rely heavily on injected services without constructors.
Default to Constructor Injection: When in doubt, favor constructor injection as it aligns with Angular's recommended practices and enhances code readability.
Conclusion
In summary, while injecting services without constructors using the inject() function is a valuable capability in Angular, it should be applied thoughtfully and sparingly, ensuring that it serves a specific purpose and doesn't compromise code maintainability or testability.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
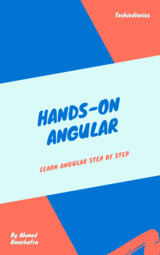