View Transitions API in angular 17 tutorial

Angular 17 introduces the View Transitions API, a game-changing feature that enables you to craft seamless and captivating animations when transitioning between pages within your application. It accomplishes this by capturing snapshots of both the current view and the incoming view and then orchestrating an animated transition between the two. This empowers you to create intricate animations without the need for custom CSS or JavaScript coding.
To harness the View Transitions API's potential, you must first import the startViewTransition function from the @angular/core package. Subsequently, you can invoke this function within your component's ngOnInit hook. startViewTransition accepts two arguments: the old view and the new view.
Here's a straightforward example of implementing the View Transitions API:
import { Component, OnInit } from '@angular/core';
import { startViewTransition } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
constructor() {}
ngOnInit(): void {
startViewTransition(this.oldView, this.newView);
}
}
In this example, oldView and newView represent references to the current view and the incoming view, respectively. The startViewTransition function orchestrates the animation between these two views.
The View Transitions API provides the flexibility to create various animations. You can achieve effects like fading between views, sliding views in from the left or right, or even crafting more intricate animations, such as a rotating cube.
For more sophisticated animations, you can utilize the startViewTransition function with the transition option. This option enables you to specify a CSS transition that will be employed for animating between the two views.
Here's an example illustrating the usage of the transition option to create a fade-in animation:
import { Component, OnInit } from '@angular/core';
import { startViewTransition } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
constructor() {}
ngOnInit(): void {
startViewTransition(this.oldView, this.newView, {
transition: 'opacity 1s ease-in-out'
});
}
}
In this instance, the transition option is configured with opacity 1s ease-in-out. Consequently, the new view gracefully fades in over a 1-second duration, utilizing an ease-in-out easing function.
The View Transitions API is a potent addition to Angular 17, enabling the creation of fluid and captivating animations during page navigation. Its ease of use and versatility empower developers to craft a wide range of animations.
Additional Tips for Leveraging the View Transitions API:
You can utilize the transition option to specify multiple CSS transitions. For instance, you can create a fading and rotating effect simultaneously. The startViewTransition function can animate between any two DOM elements, not limited to components. This means you can use the View Transitions API to animate other elements in your application, such as modals, dropdowns, and menus. While the View Transitions API is still evolving, it is generally stable and suitable for production applications. In conclusion, the View Transitions API in Angular 17 is a powerful tool that enhances page navigation animations. Its simplicity and versatility make it an attractive choice for creating engaging user experiences. If you're seeking to elevate your application's visual appeal, the View Transitions API is worth exploring.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
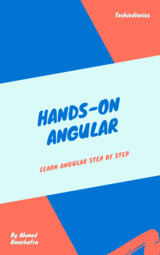