Angular 13 rating form example with Ng-Bootstrap
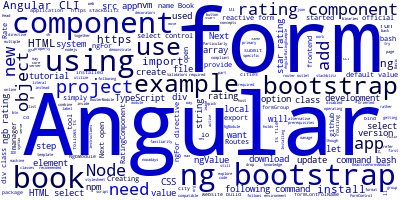
We'll learn how to integrate and employ bootstrap 5 with Angular 13 by developing an example rating application step by step.
In this part, we'll demonstrate how to use Bootstrap 5, HTML Select, and Angular 13 Forms to build a rating component. The ngb-rating
component from ng-bootstrap
will be used here. In a reactive form, we'll explore how to combine the HTML select control with the ngFor
directive. Using [ngValue]
and value
attributes, you can bind a select element to a TypeScript object or string literal, and you may provide a default value to pick from an array of items.
If you're looking for an alternative to Bootstrap UI components, go no further than NgBoostrap. Ng Bootstrap is an excellent tool for integrating bootstrap into an Angular project and using its fantastic UI components. Bootstrap has been tested and shown to work on a wide variety of devices and screen sizes. Furthermore, it has been nearly universally accepted as an industry standard.
The majority of web applications need the use of HTML forms. It is possible to utilize selects in forms if you want users to pick one of a certain number of alternatives before they can submit the form. Objects may be used as option values in Angular, rather from just strings.
Using the select control, here's an example of a component template:
<form [formGroup]="myForm">
<select formControlName="myControl">
<option [value]="city" *ngFor="let city of cities">
</option>
</select>
</form>
In our Angular component, we need to have a cities
array with some cities.
We use the value
property to bind the city to select
but you can also use ngValue
instead. The value
property is used with string literals only, whereas ngValue
can be used with objects. We'll see next another example of using select
with objects instead of strings.
This comes handy if we have a drop-down where we need to show the names of the objects from a TypeScript array. But when selecting the element from the drop-down you need to select the id
of the array element for querying the database for example. In this case, we need to use ngValue
as it works with TypeScript objects and not just strings.
You learned how to use npm to install Angular 13 CLI in the last lesson, so let's get started by starting a new project.
Prerequistes
Before getting started you need a few prerequisites:
- Basic knowledge of TypeScript. Particularly the familiarity with Object Oriented concepts such as TypeScript classes and decorators.
- A local development machine with Node 10+, together with NPM 6+ installed. Node is required by the Angular CLI like the most frontend tools nowadays. You can simply go to the downloads page of the official website and download the binaries for your operating system. You can also refer to your specific system instructions for how to install Node using a package manager. The recommended way though is using NVM — Node Version Manager — a POSIX-compliant bash script to manage multiple active Node.js versions.
Note: If you don't want to install a local environment for Angular development but still want to try the code in this angular tutorial, you can use Stackblitz, an online IDE for frontend development that you can use to create an Angular project compatible with Angular CLI.
Creating a New Angular 13 Project
Let's get started by creating a new Angular 13 project using the following command:
$ ng new AngularRatingExample
? Would you like to add Angular routing? Yes
? Which stylesheet format would you like to use? CSS
Adding Ng-Bootstrap
We’ll see how to set up Bootstrap in our project for styling our UI using ng-bootstrap
. For that purpose, we’ll first need to add bootstrap
and ng-bootstrap
from npm in our project using the following command:
$ cd AngularRatingExample
$ ng add @ng-bootstrap/ng-bootstrap
This will install ng-bootstrap
for the default application specified in your angular.json
file.
Since ng-bootstrap
has a dependency on i18n, we’ll also need add the package to our project using the following command:
$ ng add @angular/localize
Next, open the src/app/app.module.ts
file and add NgbModule
and ReactiveFormsModule
in the imports
array of AppModule
as follows:
// [...]
import { ReactiveFormsModule } from '@angular/forms';
import { NgbModule } from '@ng-bootstrap/ng-bootstrap';
@NgModule({
imports: [
// [...]
ReactiveFormsModule,
NgbModule
],
})
Next, open the src/app/app.component.html
file and update it as follows:
<div class="container">
<div class="row">
<div class="col-12">
<router-outlet></router-outlet>
</div>
</div>
</div>
We simply wrap the router outlet with some HTML markup styled with Bootstrap 5.
Creating an Angular 13 Rating Component
Next, let's create an Angular 13 component that will encapsulate our form. Head back to your terminal and run the following command:
$ ng generate component rating
Using the HTML Select Control with the ngFor
Directive with a Reactive Form
Next, open the src/app/rating/rating.component.html
file and add the following form:
<form [formGroup]="form" (ngSubmit)="submit()">
<div class="form-group">
<label>Book</label>
<select formControlName="book">
<option *ngFor="let book of books" [ngValue]="book"></option>
</select>
</div>
<div class="form-group">
<ngb-rating [max]="5" formControlName="rating"></ngb-rating>
</div>
<button [disabled]="form.invalid || form.disabled" class="btn btn-primary">Rate the book!</button>
</form>
We used the HTML select element with ngValue
, and the ngFor
directive inside our reactive form.
*ngFor
is the Angular repeater directive. It simply repeats the host element for each element in a list.
The syntax in this example is as follows:
-
<option>
is the host element. -
books
holds the books list from theRatingComponent
class. -
book
holds the current book object for each iteration through the list.
Next, update the src/app/rating/rating.component.ts
file as follows:
// [...]
export class RatingComponent implements OnInit {
books = [
{ name: 'Book 1' },
{ name: 'Book 2' },
{ name: 'Book 3' },
{ name: 'Book 4' },
{ name: 'Book 5' }
];
form = new FormGroup({
book: new FormControl(this.books[0], Validators.required),
rating: new FormControl('', Validators.required),
});
submit() {
console.log(JSON.stringify(this.form.value));
this.form.reset();
}
}
We also provided a default value from the array books for select using book: new FormControl(this.books[0], Validators.required)
. The first parameter is the default value.
Next, we need to add our rating component to the router configuration. Open the src/app/app-routing.module.ts
file and update it as follows:
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { RatingComponent } from '../rating/rating.component.ts';
const routes: Routes = [
{path: '', component: RatingComponent}
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
Finally, you can start your development server using the following command:
$ ng serve
Summary
We built a simple form with a rating component based on the ngb-rating
component from ng-bootstrap
in this Angular 13 tutorial. We've seen how to utilize the HTML select control in conjunction with the ngFor
directive inside a reactive form. How to bind select to a TypeScript object rather than a string, as well as how to provide a default value for select.
Angular 13 Star Rating Example
In this section, we'll build a star rating component with the latest Angular 13 version and Bootstrap.
Star rating is a common feature in product recommendation and eCommerce websites
We commonly rate something between zero and five stars.
Before getting started you need a few prerequisites:
- Basic knowledge of TypeScript. Particularly the familiarity with Object Oriented concepts such as TypeScript classes and decorators.
- A local development machine with Node 10+, together with NPM 6+ installed. Node is required by the Angular CLI like the most frontend tools nowadays. You can simply go to the downloads page of the official website and download the binaries for your operating system. You can also refer to your specific system instructions for how to install Node using a package manager. The recommended way though is using NVM — Node Version Manager — a POSIX-compliant bash script to manage multiple active Node.js versions.
Note: If you don't want to install a local environment for Angular development but still want to try the code in this tutorial, you can use Stackblitz, an online IDE for frontend development that you can use to create an Angular project compatible with Angular CLI.
Step 1 — Installing Angular CLI 13
Let's begin by installing the latest Angular CLI 13 version (at the time of writing this tutorial).
Angular CLI is the official tool for initializing and working with Angular projects. To install it, open a new command-line interface and run the following command:
$ npm install -g @angular/cli
At the time of writing this tutorial, angular/cli v13 will be installed on your system.
Step 2 — Creating a New Angular 13 App
In the second step, let's create our project. Head back to your command-line interface and run the following commands:
$ cd ~
$ ng new angular13star-rating
The CLI will ask you a couple of questions — If Would you like to add Angular routing? Type y for Yes and Which stylesheet format would you like to use? Choose CSS.
Next, navigate to you project’s folder and run the local development server using the following commands:
$ cd angular13star-rating
$ ng serve
Open your web browser and navigate to the http://localhost:4200/
address to see your app running.
Step 3 — Installing Ng-Bootstrap
Next, we need to install ng-bootstrap
using the following command:
$ ng add @ng-bootstrap/ng-bootstrap
This library provides an Angular implementation for Bootstrap 5 and also provides some useful components such as NgbRating -- a directive that allows you to display star rating bar.
Open the src/app/app.component.ts
file and update it as follows:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
currentRate = 0;
}
Next, open the src/app/app.component.html
file and update it as follows:
<h1 class="text-primary">
Angular 13 Start Rating Example
</h1>
<ngb-rating [max]="5" [(rate)]="currentRate" [readonly]="false"></ngb-rating>
<p>Rate: </p>
Next, open the src/app/app.component.css
file and add the following CSS styles:
ngb-rating {
font-size: 100px;
color:brown;
background: rgba(23, 221, 16, 0.815);
}
You can find this example in https://stackblitz.com/edit/angular-10-star-rating-example
Summary
In this short article, we've seen how to create a star rating component with Angular 10 and ng-bootstrap
. Read the official docs for more details.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date: