Angular Signals & Forms

Angular Signals, introduced in Angular 16, offer a revolutionary approach to form development within the Angular framework. Signals present a straightforward yet potent means of managing state and facilitating change detection in Angular applications.
One of the most compelling advantages of employing Signals for forms lies in their ability to optimize code efficiency and performance. Signals are lazily evaluated, recalculating only when their dependencies change. This feature can significantly enhance the performance of applications, especially those featuring intricate forms.
Signals also contribute to improved code readability and maintainability. They provide a concise and lucid means of expressing application logic while offering clarity regarding the interconnectedness of different application parts.
To implement Signals for forms, the initial step involves creating a signal for each piece of state that requires management. Computed signals, relying on state signals, are then created using the computed operator. Computed signals automatically update themselves when their dependencies undergo change.
To bind a Signal to a form control, the value and valid properties of the Signal are utilized. The value property retains the current Signal value, while the valid property signifies its validity.
Here's a basic example of Signals in a form context:
import { Signal } from '@angular/core';
export class MyFormComponent {
name: Signal<string> = new Signal('');
constructor() {
// Create a computed signal that depends on the name signal.
this.fullName = this.computed(() => {
return this.name.value + ' ' + 'Doe';
});
}
}
In the template for the MyFormComponent, the fullName signal can be bound to an input element. Any input by the user into the input element will automatically update the fullName signal. Angular takes care of updating the input element's value to match the new signal value.
Signals also serve as a valuable tool for form validation. For instance, a computed signal can be created to validate the name signal:
export class MyFormComponent {
name: Signal<string> = new Signal('');
// Create a computed signal that validates the name signal.
nameIsValid: Signal<boolean> = this.computed(() => {
return this.name.value.length > 0;
});
}
Subsequently, the nameIsValid signal can be bound to a div element to display a validation error message when the name is invalid.
Signals are an evolving feature in Angular, but they offer immense potential to reshape the way we approach form development.
Advantages of Using Signals for Forms
Efficiency and Performance: Signals are lazily evaluated, recalculating only when dependencies change, leading to significant performance improvements in complex forms.
Readability and Maintainability: Signals provide a clear and concise way to express application logic, making it easier to understand the connections between different parts of the application.
Flexibility: Signals can be employed for a wide array of form features, including validation, error handling, and data binding.
Conclusion
In conclusion, Angular Signals are an emerging reactive paradigm that holds the promise of transforming the way we handle forms in Angular applications. They offer efficiency, performance, and ease of use. If you're seeking a way to enhance your Angular forms, consider exploring the capabilities of Signals.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
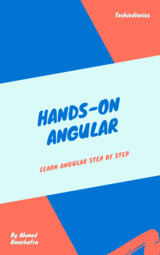