RxJS Buffer: An Effective Tool for Data Grouping and Filtering

The RxJS buffer operator stands as a potent tool for the organization and refinement of data. It provides the ability to gather emitted values into an array until a specified notifier triggers. This operator finds utility in various scenarios, including implementing caching strategies, optimizing API call frequency, and mitigating noisy data fluctuations.
In this blog post, we will delve into the buffer operator and illustrate its application in addressing common challenges within Angular applications.
Understanding the Buffer Operator:
The buffer operator operates by accumulating emitted values into an array until a designated notifier emits a signal. This notifier can take various forms, such as a timer, a user action, or any event suitable for signaling the collection of data.
Upon the notifier emitting, the buffer operator dispatches an array containing all the accumulated values. Subsequently, the operator resets and recommences the data collection process.
How to Employ the Buffer Operator:
To harness the buffer operator effectively, you must specify two crucial elements:
The number of values to accumulate before emitting an array. The notifier responsible for triggering the data collection process. The buffer operator can be applied in diverse ways, including:
- Implementing a caching mechanism.
- Reducing the frequency of API calls.
- Smoothing out erratic data fluctuations.
Example: Caching Data with the Buffer Operator:
The following example demonstrates the usage of the buffer operator to implement a caching strategy:
import { fromEvent } from 'rxjs';
import { buffer } from 'rxjs/operators';
const cache = new Map<string, any>();
const getCachedData = async (key) => {
const cachedData = cache.get(key);
if (!cachedData) {
// Perform the API call and store the results in the cache.
const apiResponse = await fetch('https://api.example.com/slow-api-call');
const data = await apiResponse.json();
cache.set(key, data);
// Return the API response.
return data;
}
// Return the cached data.
return cachedData;
};
// Utilize the buffer operator to cache API call results every 1000 milliseconds.
const cachedData = getCachedData('my-key').pipe(buffer(1000));
// Subscribe to the cached data observable.
cachedData.subscribe((data) => {
// Utilize the cached data.
});
In this illustration, the buffer operator is employed to gather API call results at 1000-millisecond intervals. Consequently, the API call is executed only once every 1000 milliseconds, regardless of the number of subscriptions to the observable.
Example: Reducing API Calls with the Buffer Operator:
The subsequent example showcases the utilization of the buffer operator to minimize the frequency of API calls:
import { fromEvent } from 'rxjs';
import { buffer } from 'rxjs/operators';
const apiCall = fromEvent(document, 'click');
// Employ the buffer operator to limit API calls to once per second.
const bufferedApiCall = apiCall.pipe(buffer(1000));
// Subscribe to the buffered API call observable.
bufferedApiCall.subscribe(() => {
// Initiate the API call.
});
In this scenario, the buffer operator is employed to accumulate clicks on the document every 1000 milliseconds. Consequently, an API call is executed only once per second, even if the user clicks multiple times consecutively.
Example: Smoothing Noisy Data with the Buffer Operator:
The subsequent example demonstrates how to utilize the buffer operator to mitigate data noise:
import { fromEvent } from 'rxjs';
import { buffer } from 'rxjs/operators';
const sensorData = fromEvent(sensor, 'change');
// Employ the buffer operator to smooth sensor data.
const smoothedSensorData = sensorData.pipe(buffer(100));
// Subscribe to the smoothed sensor data observable.
smoothedSensorData.subscribe((data) => {
// Utilize the smoothed sensor data.
});
In this context, the buffer operator is used to accumulate sensor data every 100 milliseconds. Consequently, updates to the smoothed sensor data are emitted only once every 100 milliseconds, even in the presence of rapid data fluctuations.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
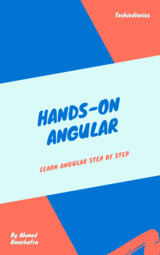